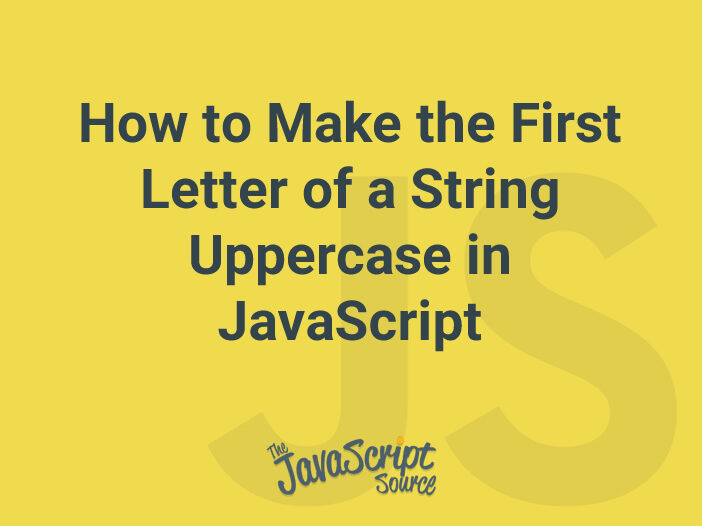
JavaScript provides several built-in methods for manipulating strings, including one called toUpperCase()
which can be used to convert the first letter of a string to uppercase.
Here is an example of how to use the toUpperCase()
method to make the first letter of a string uppercase:
let str = "hello world";
let firstLetter = str.charAt(0);
let upperFirstLetter = firstLetter.toUpperCase();
let restOfString = str.slice(1);
let newString = upperFirstLetter + restOfString;
console.log(newString); // Output: "Hello world"
In this example, the charAt(0)
method is used to get the first letter of the string and store it in the firstLetter
variable. The toUpperCase()
method is then used to convert the firstLetter
to uppercase and store it in the upperFirstLetter
variable. The slice(1)
method is then used to get the rest of the string, after the first letter, and store it in the restOfString
variable. Finally, the upperFirstLetter
and restOfString
are concatenated together to create a new string with the first letter in uppercase.
Alternatively, a one-liner solution can be achieved by using the following code snippet:
let newString = str.replace(/^\w/, c => c.toUpperCase());
console.log(newString); // Output: "Hello world"
This uses the replace function with regular expression /^\w/
which matches the first word character of the string and replace it with the uppercase version of that character.
Another way to do this is by using the substring()
and toUpperCase()
method:
let newString = str.substring(0, 1).toUpperCase() + str.substring(1);
console.log(newString); // Output: "Hello world"
This creates a new string by taking the first character of the input string and converting it to uppercase, then concatenating it with the rest of the string after the first character.
These are just a few examples of how to make the first letter of a string uppercase in JavaScript. Depending on your specific use case, one method may be more appropriate than another.