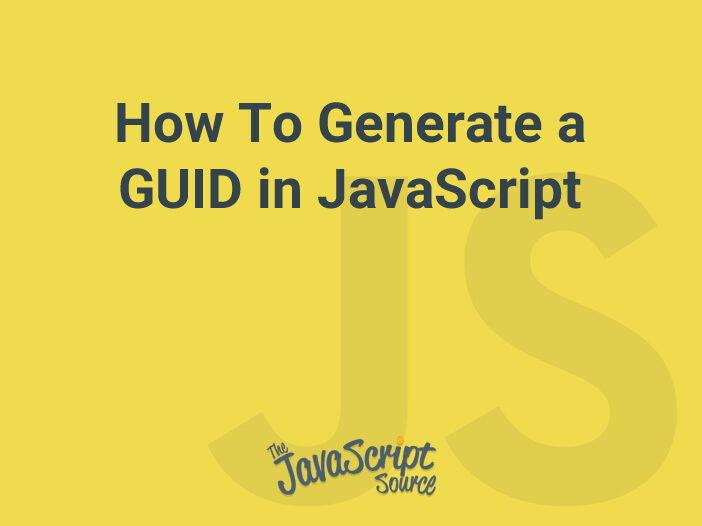
A globally unique identifier (GUID) is a 128-bit value that is used to identify resources, such as records in a database, in a unique and consistent manner across different systems. In JavaScript, there are several libraries and built-in methods that can be used to generate a GUID.
One way to generate a GUID in JavaScript is to use the crypto
module, which is built into modern web browsers and Node.js. The crypto.getRandomValues()
method generates a cryptographically-secure, random array of values that can be used to create a GUID. Here is an example of how to use the crypto
module to generate a GUID:
function generateGUID() {
const array = new Uint8Array(16);
crypto.getRandomValues(array);
array[6] = (array[6] & 0x0f) | 0x40;
array[8] = (array[8] & 0x3f) | 0x80;
let guid = '';
for (let i = 0; i < array.length; i++) {
let value = array[i].toString(16);
if (value.length === 1) {
value = '0' + value;
}
guid += value;
if (i === 3 || i === 5 || i === 7 || i === 9) {
guid += '-';
}
}
return guid;
}
Another popular library for generating GUIDs in JavaScript is the uuid
library. It is a simple, lightweight library that can be used to generate a GUID in a single line of code:
const uuid = require('uuid');
const newGUID = uuid.v4();
or
import { v4 as uuidv4 } from 'uuid';
const newGUID = uuidv4();
Both of these methods will generate a unique GUID that can be used to identify resources in a consistent and unique manner. You can choose which method you prefer or which fits your use case best.