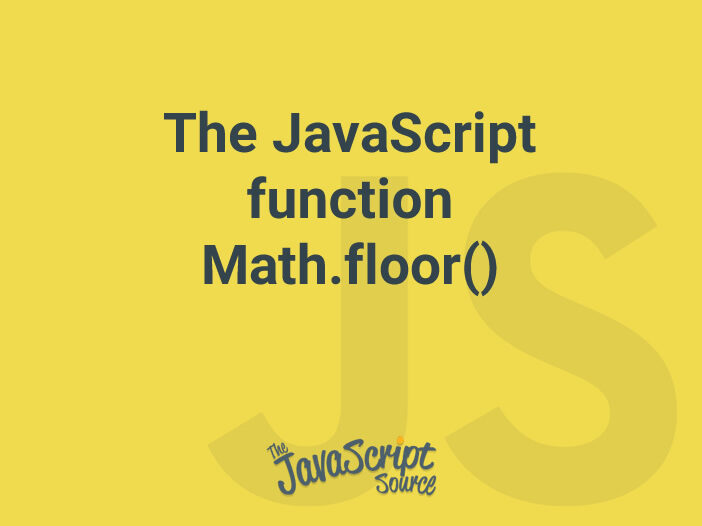
The JavaScript function Math.floor()
is used to round a number down to the nearest integer. This function takes a single argument, which is the number that you want to round down.
For example, if you wanted to round the number 4.7 down to the nearest integer, you would use the following code:
let number = 4.7;
let rounded = Math.floor(number);
console.log(rounded); // Output: 4
As you can see, the Math.floor()
function is called with the variable number
as the argument, and the result is stored in the variable rounded
. When you run this code, the output will be 4, which is the nearest integer less than 4.7.
You can also use Math.floor()
to round down negative numbers. For example:
let negativeNumber = -4.7;
let rounded = Math.floor(negativeNumber);
console.log(rounded); // Output: -5
In this example, the number -4.7 is passed to the Math.floor()
function, and the result is -5, which is the nearest integer less than -4.7.
Additionally, you can use the Math.floor()
function with expressions, for example:
let number = 5 / 2;
let rounded = Math.floor(number);
console.log(rounded); // Output: 2
The Math.floor()
function is a useful tool for working with numbers in JavaScript, and it can be used in a variety of situations where you need to round a number down to the nearest integer.