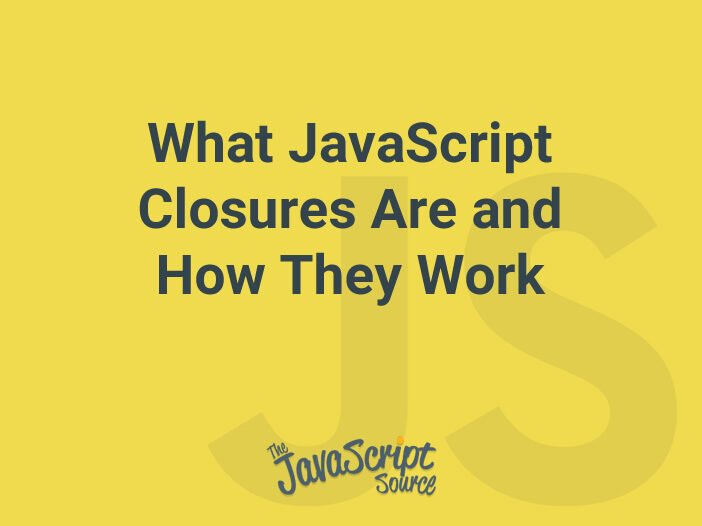
JavaScript closures are a fundamental concept in the JavaScript programming language. A closure is a function that has access to the variables in the scope in which it was created, even after the function has executed and the scope in which it was created no longer exists.
Here’s an example of a closure:
function outerFunction(x) {
return function innerFunction(y) {
return x + y;
}
}
let add5 = outerFunction(5);
console.log(add5(3)); // 8
In this example, the outerFunction
returns the innerFunction
, which has access to the x
variable. Even though the outerFunction
has finished executing, the innerFunction
still has access to the x
variable and can use it in its own execution.
In the last line, the outerFunction
is called with an argument of 5
, which is passed to x
, so the returned innerFunction
has access to x
as 5
. Then we store the returned innerFunction
in a variable called add5
and call it with an argument 3
which is passed to y
, and return the value of x+y
which is 8
.
Here’s another example that shows how closures can be used to create private variables in JavaScript:
function createCounter() {
let count = 0;
return {
increment: function() {
count++;
},
decrement: function() {
count--;
},
getCount: function() {
return count;
}
}
}
let counter = createCounter();
console.log(counter.getCount()); // 0
counter.increment();
console.log(counter.getCount()); // 1
counter.decrement();
console.log(counter.getCount()); // 0
In this example, the createCounter
function creates an object with three methods: increment
, decrement
, and getCount
. The count
variable is defined within the scope of the createCounter
function, but is still accessible to the methods because they are closures. The methods can access and modify the count
variable, but it is not directly accessible from outside the createCounter
function.