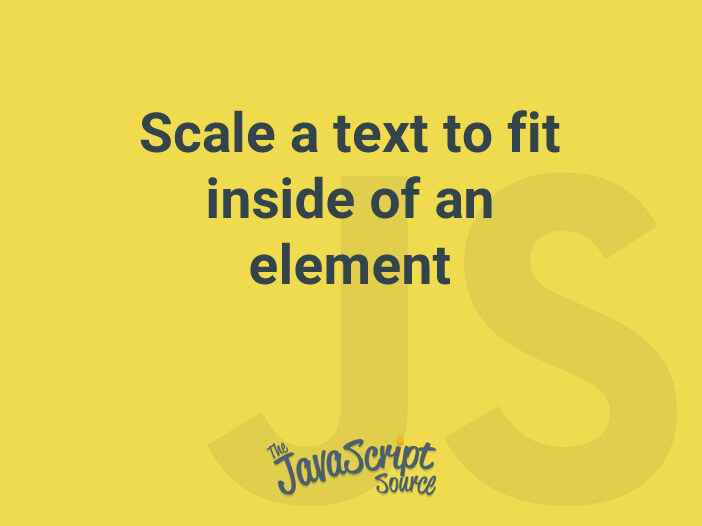
Let’s say that we want to scale a text inside a headline:
<div id="headline">Hello World</div>
First of all, we need to measure the width of element with its current font size and text content.
const measureWidth = function(text, font) {
// Measure the width of given text for given font
...
};
// Query the element
const ele = document.getElementById('headline');
// Get the styles
const styles = window.getComputedStyle(ele);
// Get the font size and font style
const font = styles.font;
const fontSize = parseInt(styles.fontSize);
const measured = measureWidth(ele.textContent, font);
Now we can calculate how much the element is scaled by comparing the measured width and the full width:
const scale = ele.clientWidth / parseFloat(measured);
Finally, we set the font size as the element scales up to full width:
const scaleFontSize = Math.floor(scale * fontSize);
ele.style.fontSize = `${scaleFontSize}px`;
Source
https://htmldom.dev/scale-a-text-to-fit-inside-of-an-element/