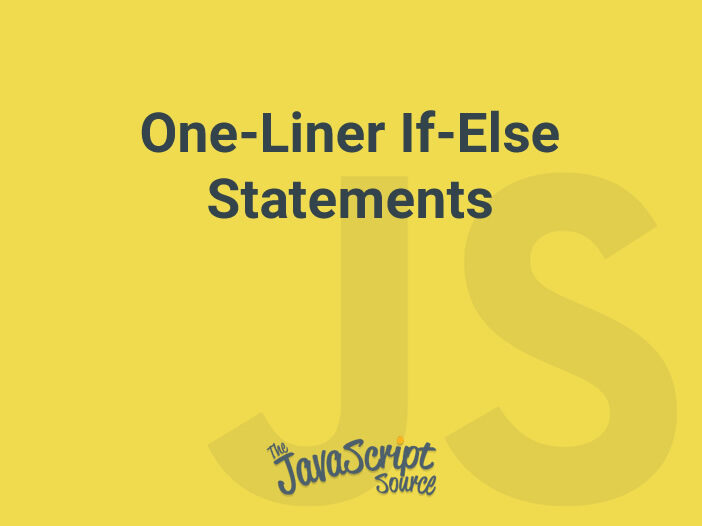
This is a common feature in many programming languages. Instead of writing an if-else on multiple lines, you can use the ternary operator to write the whole statement with one line of code.
For example:
const age = 12;
let ageGroup;
// LONG FORM
if (age > 18) {
ageGroup = "An adult";
} else {
ageGroup = "A child";
}
// SHORTHAND
ageGroup = age > 18 ? "An adult" : "A child";
However, do not overuse this. It can quickly make your code more verbose too. It is wise to replace only simple expressions with this to improve readability and reduce lines of code.
Source
https://medium.com/geekculture/20-javascript-snippets-to-code-like-a-pro-86f5fda5598e