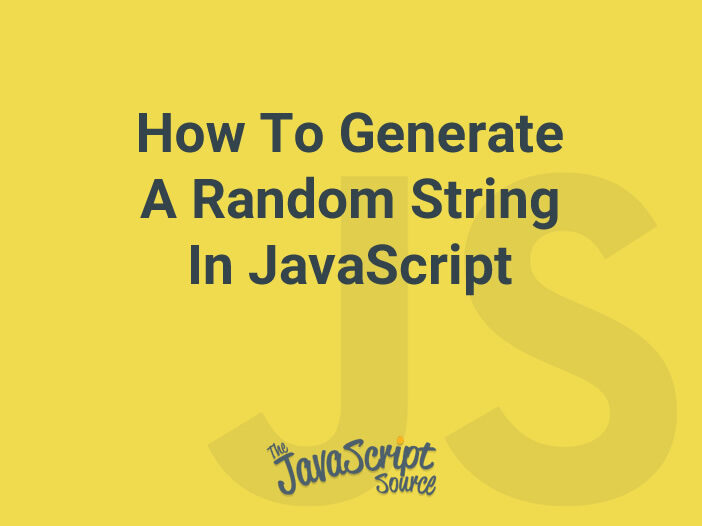
Generating a random string in JavaScript can be useful for a variety of tasks, such as generating unique identifiers or password reset tokens. There are a few different ways to generate a random string in JavaScript, but one of the simplest is to use the built-in Math.random()
function.
Here is an example of how to use Math.random()
to generate a random string of a specified length:
function generateRandomString(length) {
let result = '';
const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
const charactersLength = characters.length;
for (let i = 0; i < length; i++) {
result += characters.charAt(Math.floor(Math.random() * charactersLength));
}
return result;
}
console.log(generateRandomString(5)); // example output: "g5X8y"
In this example, the generateRandomString
function takes a single argument, length
, which specifies the length of the random string to generate. The function then declares a variable result
which is initially set to an empty string. Next, it declares a string characters
containing all the characters that can be used in the random string.
The function then uses a for loop to iterate length
number of times. In each iteration, it uses Math.random()
to generate a random number between 0 and 1, and then multiplies that number by the length of the characters
string. This results in a random index between 0 and the length of the characters
string. The charAt()
method is then used to retrieve the character at that index in the characters
string, and the result is added to the result
string.
Finally, the result
string is returned.
You can also use the crypto
library to generate a random string. This is particularly useful for cryptographic purposes.
function generateRandomString(length) {
let result = '';
const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
const charactersLength = characters.length;
const crypto = window.crypto || window.msCrypto;
const array = new Uint8Array(length);
crypto.getRandomValues(array);
array.forEach(x => {
result += characters[x % charactersLength];
});
return result;
}
console.log(generateRandomString(5));
In this approach, we use the crypto.getRandomValues()
method to generate a random number of bytes, then use the modulo operator %
on the value of each byte and the length of characters string to get the corresponding character to concatenate it to the result string.
In both examples, you can change the characters string to suit your needs, for example you can remove characters that you don’t want to include in the string or add more characters to the set.