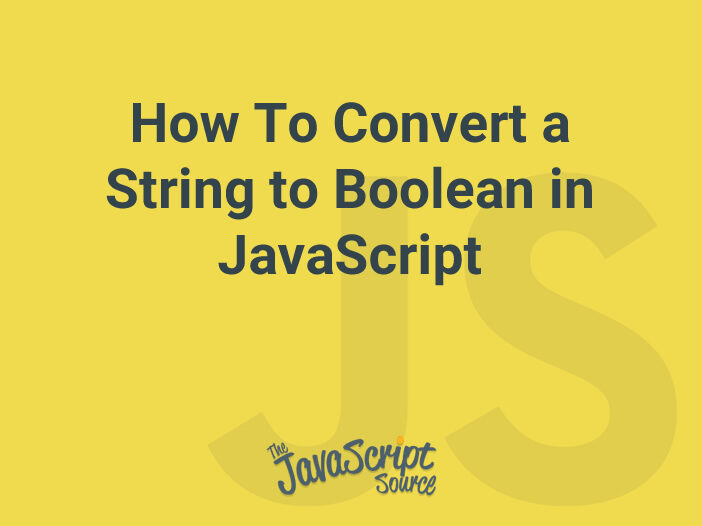
JavaScript has several ways to convert a string to a boolean. One way is to use the Boolean()
function, which returns the boolean value of a given variable. For example:
let str = "true";
let bool = Boolean(str);
console.log(bool); // outputs: true
Another way is to use the ==
or ===
operator to compare the string to the boolean values true
or false
. The ==
operator performs type coercion, meaning it will try to convert the string to a boolean before comparing it. The ===
operator, on the other hand, does not perform type coercion and compares the string and boolean values directly.
let str = "true";
let bool = (str == true);
console.log(bool); // outputs: true
let str = "true";
let bool = (str === true);
console.log(bool); // outputs: false
Another way to convert string to boolean is using JSON.parse()
method which parses a string as JSON and returns JavaScript objects.
let str = "true";
let bool = JSON.parse(str);
console.log(bool); // outputs: true
You can also use if(str)
statement. In this case if the string is “truthy” it will return true, otherwise false.
let str = "true";
if(str) {
console.log(true);
}
else {
console.log(false);
} // outputs: true
In JavaScript, the following values are considered “falsy”:
false
0
(zero)""
(empty string)null
undefined
NaN
So, if you use if(str)
statement, it will return true for any string which is not “falsy”.
It is important to note that when using the ==
operator, the string “false” will be considered true because it is not one of the falsy values listed above. To avoid this, it is best to use the ===
operator or the Boolean()
function when converting strings to booleans in JavaScript.