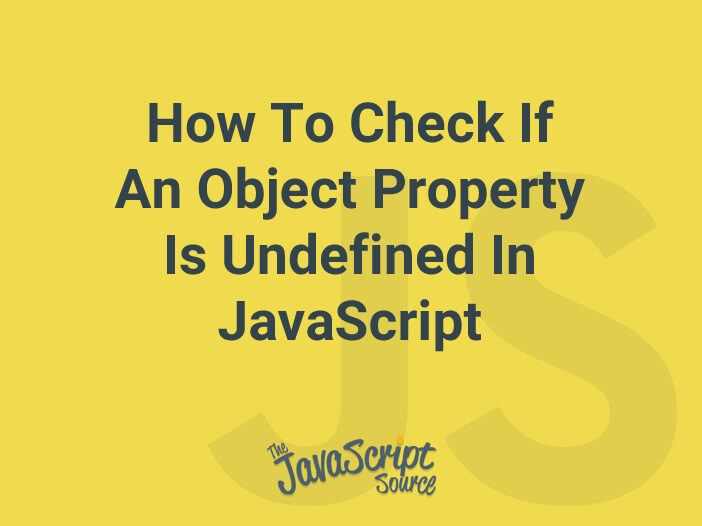
JavaScript provides several ways to check if an object property is undefined. One way is to use the typeof
operator, which returns the type of a variable or an expression. When used with an object property, it returns “undefined” if the property does not exist or has not been assigned a value. Here is an example:
let myObject = { prop1: "value1" };
console.log(typeof myObject.prop2); // "undefined"
Another way to check if an object property is undefined is to use the in
operator, which returns a Boolean value indicating whether an object has a given property. Here is an example:
let myObject = { prop1: "value1" };
console.log("prop2" in myObject); // false
A third way to check if an object property is undefined is to use the hasOwnProperty()
method, which returns a Boolean value indicating whether an object has a given property. Here is an example:
let myObject = { prop1: "value1" };
console.log(myObject.hasOwnProperty("prop2")); // false
You can also use the undefined
keyword to check if a property is undefined. Here is an example:
let myObject = { prop1: "value1" };
console.log(myObject.prop2 === undefined); // true
console.log(myObject.prop1 === undefined); // false
In JavaScript, it’s also a common practice to use the ==
operator to check if a property is undefined. However, this approach can lead to unexpected results when the property value is null
or NaN
.
let myObject = { prop1: "value1", prop2:null };
console.log(myObject.prop2 == undefined); // true
console.log(myObject.prop1 == undefined); // false
It is recommended to use the ===
operator instead of ==
operator in order to avoid unexpected results.