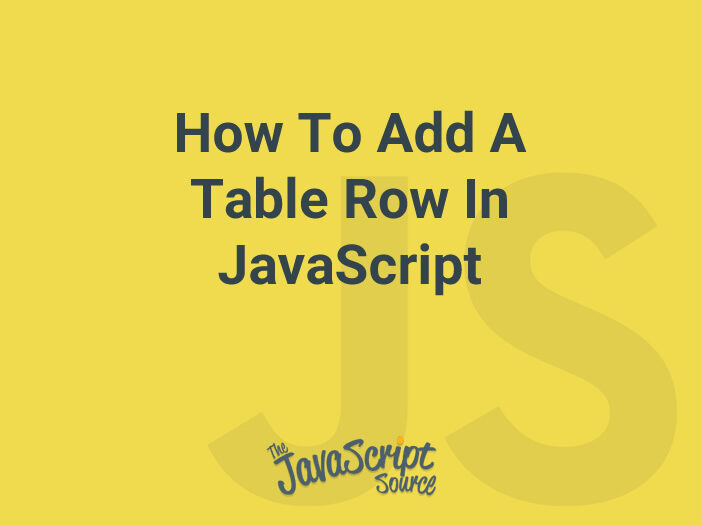
Adding a table row in JavaScript can be done using the DOM (Document Object Model) API. The DOM API allows you to manipulate the elements of an HTML or XML document, including table rows.
To add a table row in JavaScript, you first need to find the table element in the document. This can be done using the document.getElementById()
method, which returns the element with the specified ID. Once you have a reference to the table element, you can create a new row element using the document.createElement()
method. Then, you can add cells to the row using the appendChild()
method.
Here is an example of how to add a table row to a table with the ID “myTable”:
// Get the table element
var table = document.getElementById("myTable");
// Create a new row element
var newRow = document.createElement("tr");
// Create cells
var cell1 = document.createElement("td");
var cell2 = document.createElement("td");
// Add text to cells
cell1.innerHTML = "Column 1";
cell2.innerHTML = "Column 2";
// Add cells to row
newRow.appendChild(cell1);
newRow.appendChild(cell2);
// Add row to table
table.appendChild(newRow);
In the example above, we first find the table element with the ID “myTable”. Then, we create a new row element and two cell elements. We add text to the cells using the innerHTML
property, and append the cells to the row using the appendChild()
method. Finally, we append the row to the table using the appendChild()
method.
This is just a basic example of how to add a table row in JavaScript. You can also add more cells to the row and customize the content of the cells as needed. Additionally, you can also use JavaScript to add rows to the table dynamically in response to user interactions or other events.
Note: The above code will work when JavaScript is executed after the page load. In case you need to run the script before the page load you should make use of the DOMContentLoaded event, like this:
document.addEventListener("DOMContentLoaded", function(){
// Get the table element
var table = document.getElementById("myTable");
// Create a new row element
var newRow = document.createElement("tr");
// Create cells
var cell1 = document.createElement("td");
var cell2 = document.createElement("td");
// Add text to cells
cell1.innerHTML = "Column 1";
cell2.innerHTML = "Column 2";
// Add cells to row
newRow.appendChild(cell1);
newRow.appendChild(cell2);
// Add row to table
table.appendChild(newRow);
});
This way the script will wait until the DOM is loaded before manipulating it.