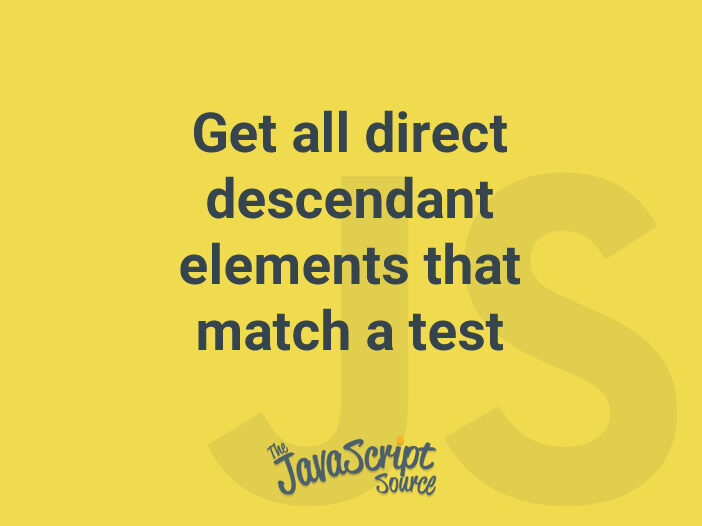
To do this, we need to combine Node.children
with a few other techniques.
We can use Array.from()
to turn the NodeList that Node.children
returns into an array, and then use the Array.filter()
method to remove an elements that don’t match a test we specify.
/*!
* Get all direct descendant elements that match a test condition
* (c) 2021 Chris Ferdinandi, MIT License, https://gomakethings.com
* @param {Node} elem The element to get direct descendants for
* @param {Function} callback The test condition
* @return {Array} The matching direct descendants
*/
function childrenMatches (elem, callback) {
return Array.from(elem.children).filter(callback);
}