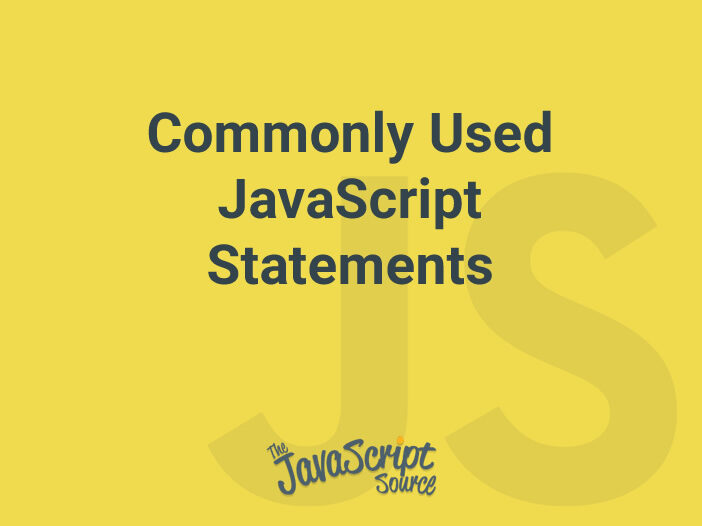
In this article, we will discuss some of the most commonly used JavaScript statements and provide code examples for each.
var
statement
The var
statement is used to declare a variable in JavaScript. Variables are used to store data, such as numbers, strings, and objects.
var x = 5;
var y = "Hello World";
var z = {name: "John", age: 30};
let
statement
Similar to var
, let
statement is also used to declare a variable in javascript, but it has a block level scope, unlike var which has a function level scope.
let x = 5;
let y = "Hello World";
let z = {name: "John", age: 30};
const
statement
const
is also used to declare a variable but it can’t be reassigned after it has been declared.
const x = 5;
const y = "Hello World";
const z = {name: "John", age: 30};
if
statement
The if
statement is used to execute a block of code if a certain condition is true.
if (x > 5) {
console.log("x is greater than 5");
}
else
statement
The else
statement is used in conjunction with the if
statement to execute a block of code if the condition in the if
statement is false.
if (x > 5) {
console.log("x is greater than 5");
} else {
console.log("x is not greater than 5");
}
else if
statement
The else if
statement is used in conjunction with the if
and else
statements to test multiple conditions.
if (x > 5) {
console.log("x is greater than 5");
} else if (x < 5) {
console.log("x is less than 5");
} else {
console.log("x is equal to 5");
}
for
statement
The for
statement is used to execute a block of code a specified number of times.
for (var i = 0; i < 10; i++) {
console.log(i);
}
while
statement
The while
statement is used to execute a block of code while a certain condition is true.
while (x < 10) {
console.log(x);
x++;
}
do...while
statement
The do...while
statement is similar to the while
statement, but the code block is executed at least once before the condition is evaluated.
do {
console.log(x);
x++;
} while (x < 10);
break
statement
The break
statement is used to exit a loop or a switch statement.
for (var i = 0; i < 10; i++) {