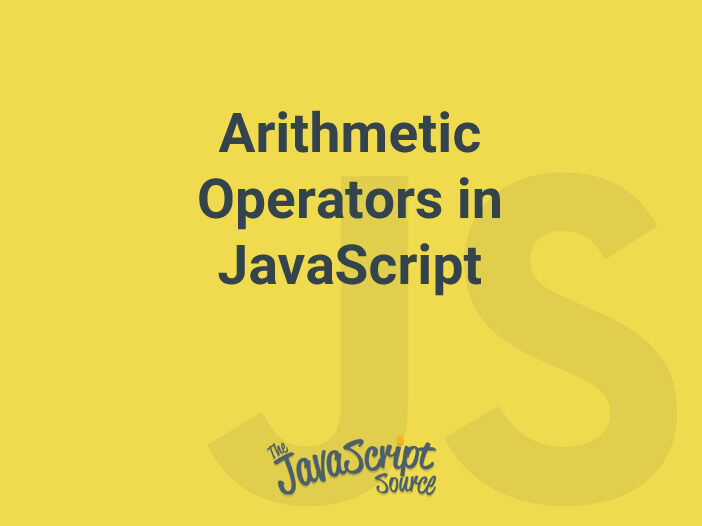
JavaScript has several arithmetic operators that are used to perform mathematical operations on numbers. These operators include:
Addition (+): This operator is used to add two or more numbers together.
2 + 3 = 5
Subtraction (-): This operator is used to subtract one number from another.
5 - 2 = 3
Multiplication (*): This operator is used to multiply two or more numbers together.
2 * 3 = 6
Division (/): This operator is used to divide one number by another.
6 / 2 = 3
Modulus (%): This operator returns the remainder of a division.
5 % 2 = 1
Increment (++): This operator is used to increase the value of a variable by 1.
ex = 5;
x++;
console.log(x); //6
Decrement (–): This operator is used to decrease the value of a variable by 1.
x = 5;
x--;
console.log(x); //4
Exponentiation (**): This operator is used to raise a number to a specified power.
2 ** 3 = 8
In JavaScript, these operators can be used in both prefix and postfix notation. In prefix notation, the operator is placed before the operand (e.g. ++x
), while in postfix notation, the operator is placed after the operand (e.g. x++
).
It’s important to note that these operators have a precedence, meaning that some of them will be executed before others. For example, multiplication and division will be executed before addition and subtraction. In case you want to change the order of operations you can use parentheses.
In addition to these basic arithmetic operators, JavaScript also has several built-in mathematical functions, such as Math.abs()
(absolute value), Math.floor()
(round down to the nearest integer), and Math.pow()
(raise a number to a specified power). These functions can be used to perform more complex mathematical operations.