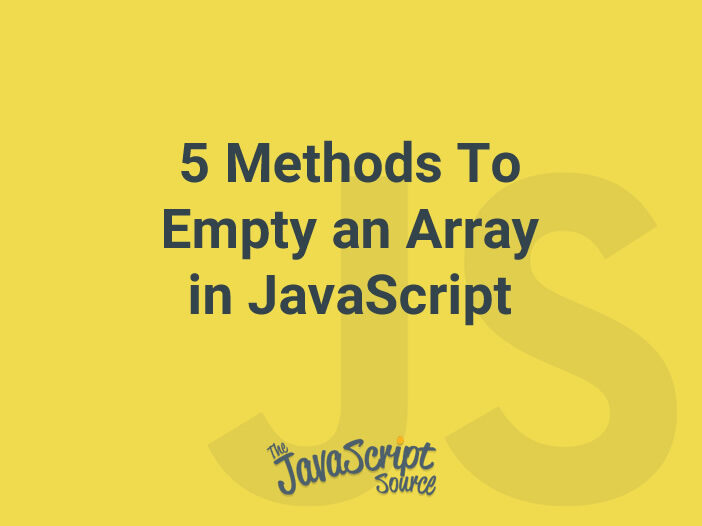
JavaScript provides several ways to empty an array. Here are a few common methods:
Using the length
property
This method sets the length of the array to 0, effectively removing all elements from the array.
let myArray = [1, 2, 3, 4, 5];
myArray.length = 0;
console.log(myArray); // []
Using the splice()
method
This method can be used to remove elements from an array by specifying the starting index and the number of elements to remove. By passing 0 as the second argument, we can remove all elements from the array.
let myArray = [1, 2, 3, 4, 5];
myArray.splice(0, myArray.length);
console.log(myArray); // []
Using the slice()
method
The slice()
method can be used to create a new array by selecting elements from an existing array. By passing 0 as the first argument and the length of the array as the second argument, we can create a new array that is a copy of the original array.
let myArray = [1, 2, 3, 4, 5];
let newArray = myArray.slice(0, myArray.length);
console.log(newArray); // [1, 2, 3, 4, 5]
Re-assigning the array variable
This method involves re-assigning the array variable to a new array.
let myArray = [1, 2, 3, 4, 5];
myArray = [];
console.log(myArray); // []
Using fill()
method
The fill()
method can be used to fill all the elements of an array with a static value. By passing 0 as the first argument and the length of the array as the second argument, we can fill all the elements with 0.
let myArray = [1, 2, 3, 4, 5];
myArray.fill(0);
console.log(myArray); // [0,0,0,0,0]
It’s important to note that methods 1, 2, and 5 will modify the original array, while methods 3 and 4 will create a new array. You can use any of these methods depending on your requirement and the situation.