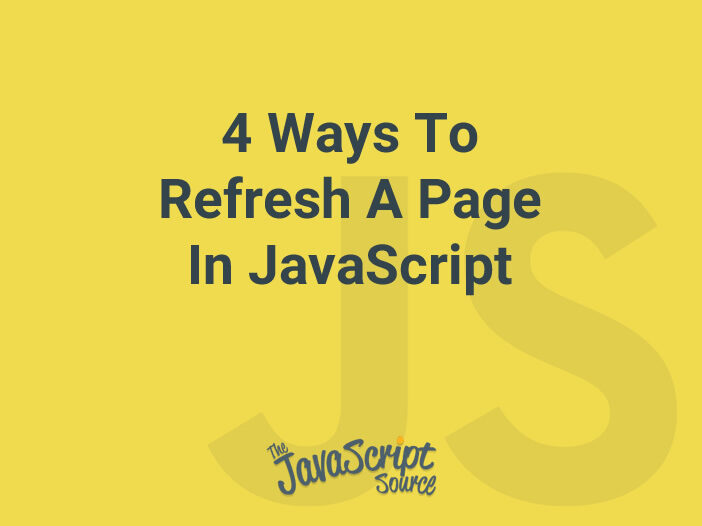
There are several ways to refresh a page using JavaScript, and in this article, we will cover the most common methods.
Method 1: Using the location.reload()
Method
The simplest way to refresh a page using JavaScript is by using the location.reload()
method. This method reloads the current page with the current URL. To use this method, you can simply call it on the location object, like so:
location.reload();
Method 2: Using the location.href
Property
Another way to refresh a page using JavaScript is by using the location.href
property. This property sets or returns the current URL of the current page. To refresh the page, you can set the location.href
property to the current URL, like so:
location.href = location.href;
Method 3: Using the location.replace()
Method
The location.replace()
method is similar to the location.href property, but it also replaces the current page in the browser history. This means that the current page will be removed from the browser’s history, and the user will not be able to use the back button to navigate to the previous page. To use this method, you can call it on the location object and pass in the current URL, like so:
location.replace(location.href);
Method 4: Using a Timer
You can also refresh a page using JavaScript by using a timer. The setTimeout()
function can be used to execute a function after a specified amount of time has passed. To refresh the page using a timer, you can use the location.reload()
method inside a function and pass that function to the setTimeout()
function, like so:
setTimeout(function(){
location.reload();
}, 5000);
This will refresh the page after 5 seconds.