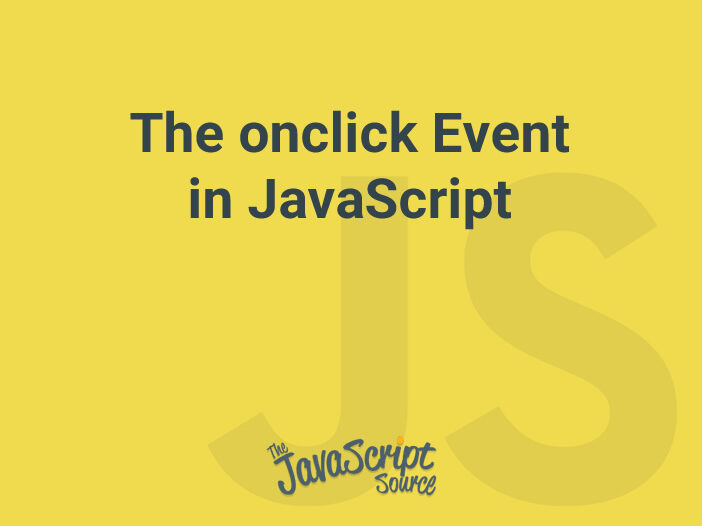
The JavaScript onclick
event is triggered when an element is clicked on, such as a button or a link. This event can be used to perform various actions, such as submitting a form, navigating to a new page, or displaying a message.
Here is an example of using the onclick
event to submit a form:
<form>
<label for="name">Name:</label>
<input type="text" id="name">
<input type="button" value="Submit" onclick="submitForm()">
</form>
function submitForm() {
document.forms[0].submit();
}
In this example, the submitForm()
function is called when the “Submit” button is clicked. The function then submits the form by calling the submit()
method on the first form on the page.
Another example of using onclick
event is when you want to navigate to a new page
<a href="#" onclick="goToPage()">Go to page</a>
function goToPage() {
window.location.href = "http://example.com";
}
In this example, when the user clicks on the “Go to page” link, the goToPage()
function is called, and the user will be redirected to “http://example.com”
You can also use onclick
event on multiple elements, for example:
<button onclick="changeColor(this)">Change color</button>
<button onclick="changeColor(this)">Change color</button>
function changeColor(el) {
el.style.backgroundColor = "red";
}
In this example, when the user clicks on any of the buttons, the changeColor()
function is called, and the background color of the button that is clicked on will change to red.
It’s important to note that onclick
event is supported by all major browsers including Internet Explorer, Google Chrome, and Mozilla Firefox.