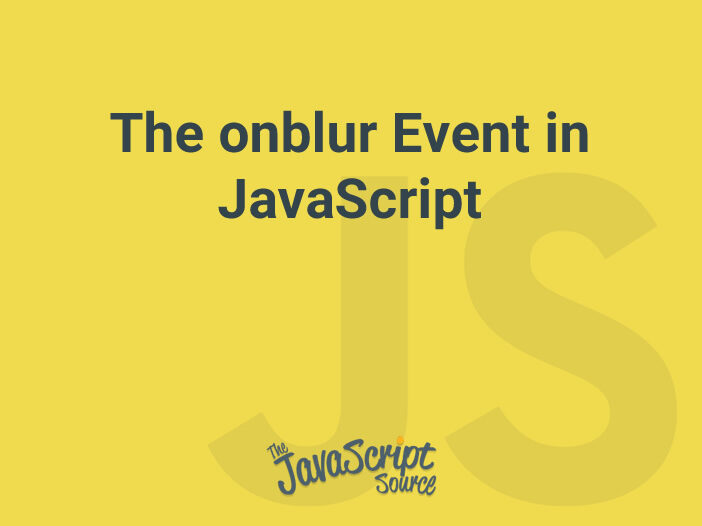
The JavaScript onblur
event is triggered when an element loses focus, such as when a user clicks outside of an input field or tabs away from a text area. This event can be useful for validating form input, hiding or showing additional content, or triggering other actions.
Here is an example of using the onblur
event to check if a form input is empty:
<form>
<label for="name">Name:</label>
<input type="text" id="name" onblur="validateName()">
<span id="nameError"></span>
</form>
function validateName() {
var name = document.getElementById("name").value;
if (name == "") {
document.getElementById("nameError").innerHTML = "Name is required.";
} else {
document.getElementById("nameError").innerHTML = "";
}
}
In this example, the validateName()
function is called when the user tabs away or clicks outside of the “Name” input field. If the input is empty, an error message is displayed next to the input field.
Another example of using onblur
event is when you want to show a message to the user when they click outside of a specific element
<div onblur="showMessage()">
<p>Click anywhere outside of this div to show a message</p>
</div>
function showMessage() {
alert("You clicked outside of the div");
}
In this example, when the user clicks outside of the div, the showMessage()
function is called, and an alert is displayed with the message “You clicked outside of the div.”
It’s important to note that onblur
event is supported by all major browsers including Internet Explorer, Google Chrome, and Mozilla Firefox.