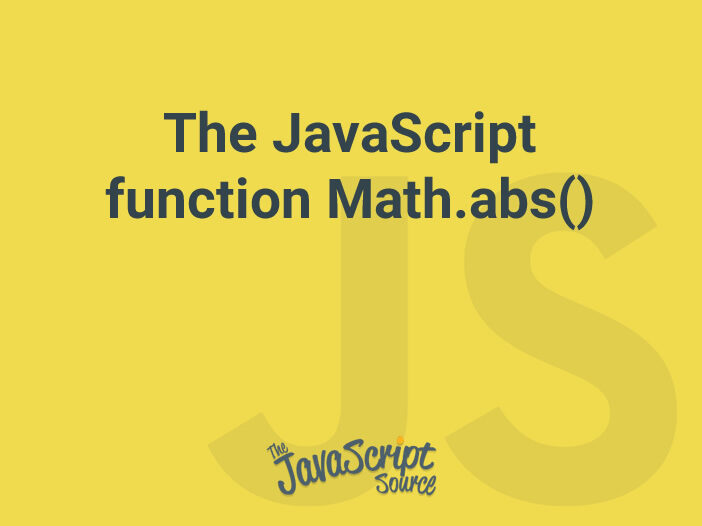
The JavaScript function Math.abs()
is a built-in function that returns the absolute value of a number. The absolute value of a number is its distance from zero, regardless of whether the number is positive or negative.
Here is an example of how to use the Math.abs()
function:
let num1 = -5;
let num2 = 5;
console.log(Math.abs(num1)); // Output: 5
console.log(Math.abs(num2)); // Output: 5
In the above example, num1
is assigned the value of -5 and num2
is assigned the value of 5. When the Math.abs()
function is called on these variables, it returns the absolute value of each number, which is 5 in both cases.
You can also use the Math.abs()
function on decimal numbers:
let num3 = -3.14;
let num4 = 3.14;
console.log(Math.abs(num3)); // Output: 3.14
console.log(Math.abs(num4)); // Output: 3.14
As you can see, the Math.abs()
function works the same way on decimal numbers as it does on whole numbers.
It is also worth noting that the Math.abs()
function does not modify the original variable and returns a new value.
The Math.abs()
function can be useful in a variety of situations, such as when you need to determine the distance between two points on a coordinate plane or when you need to calculate the magnitude of a vector.
In short, the JavaScript function Math.abs()
returns the absolute value of a number, regardless of whether it is positive or negative. It can be used on both whole numbers and decimal numbers and is a useful tool for a variety of mathematical calculations.