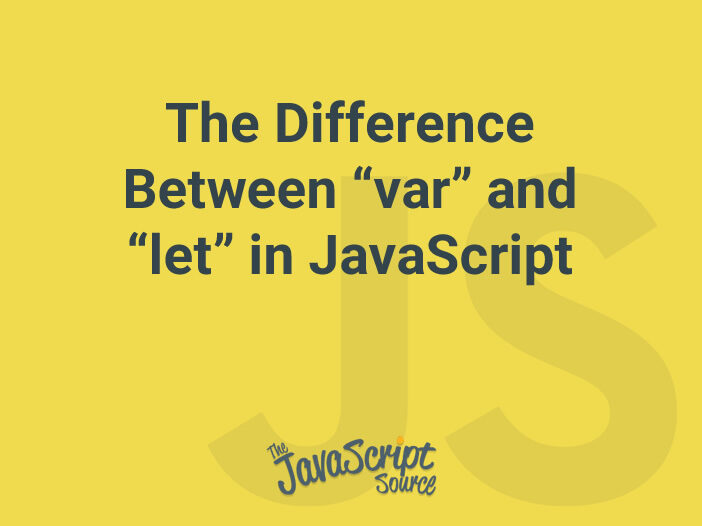
JavaScript has two ways to declare variables: var
and let
. Although they both serve the same purpose, there are some key differences between the two.
The var
keyword is used to declare a variable in JavaScript, and it has function scope. This means that a variable declared with var
is accessible within the entire function in which it is declared, and any nested functions. If a variable is declared with var
outside of any function, it becomes a global variable and can be accessed from anywhere in the code. Here’s an example:
function example() {
var x = 10;
console.log(x); // prints 10
}
example();
console.log(x); // ReferenceError: x is not defined
In this example, the variable “x” is declared within the “example” function using the var
keyword. When we call the function and print the value of “x”, it prints 10 as expected. However, if we try to access “x” outside of the function, we get a ReferenceError because “x” is only defined within the “example” function.
On the other hand, the let
keyword is also used to declare a variable in JavaScript, but it has block scope. This means that a variable declared with let
is only accessible within the block in which it is declared, and any nested blocks. Here’s an example:
let x = 10;
if (true) {
let x = 20;
console.log(x); // prints 20
}
console.log(x); // prints 10
In this example, we first declare a variable “x” with the value of 10 using the let
keyword. Then, within an if block, we declare another variable “x” with the value of 20 using the let
keyword. When we print the value of “x” within the if block, it prints 20 because the inner “x” variable shadows the outer one. However, when we print the value of “x” outside of the if block, it prints 10 because the inner “x” variable only exists within the if block.
In summary, var
has function scope and let
has block scope. The difference between these two is that var
variables are accessible within the entire function and let
variables are accessible only within the block they are declared.
It is important to note that, while var
is a keyword available in JavaScript since the start, the keyword let
was introduced in ECMAScript 6 (ES6) which is the latest version of JavaScript and it is recommended to use let
and const
for variable declaration instead of var
.