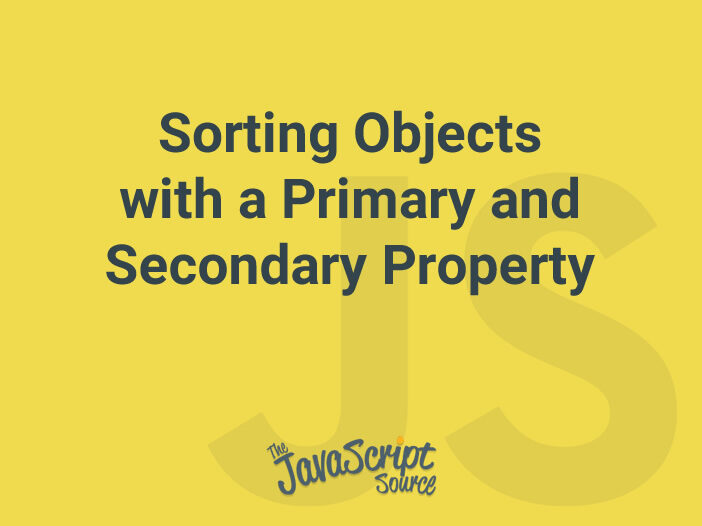
When you sort an array and have several matches exact matches, it is handy to have a secondary property to sort by to break the tie. You can use the same approach as above, but with a little more logic. Inside the compareFunction
, if the two properties have the same value (a zero compare value), then you can compare again by a secondary property.
let objects = [
{ name: "nop", value: 3 },
{ name: "NOP", value: 2 },
{ name: "ñop", value: 1 },
{ name: "abc", value: 3 },
{ name: "abc", value: 2 },
{ name: "äbc", value: 1 },
];
const collator = new Intl.Collator('en');
objects.sort((a, b) => {
// Compare the strings via locale
let diff = collator.compare(a.name, b.name);
if (diff === 0) {
// If the strings are equal compare the numbers
return a.value - b.value;
}
return diff;
});
console.log(objects);
/*
[
{ "name": "abc", "value": 2 }, // name is same, sort by value
{ "name": "abc", "value": 3 }, // name is same, sort by value
{ "name": "äbc", "value": 1 },
{ "name": "nop", "value": 3 },
{ "name": "NOP", "value": 2 },
{ "name": "ñop", "value": 1 }
]
*/