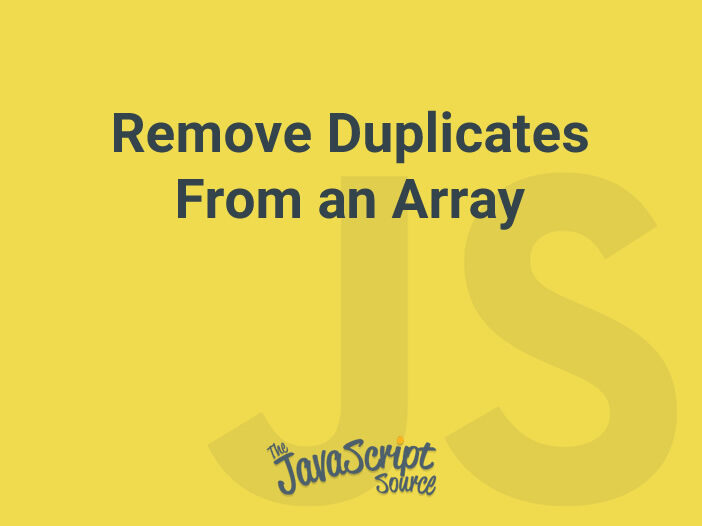
In JavaScript, Set is a collection that lets you store only unique values. This means any duplicated values are removed.
So, to remove duplicates from an array, you can convert it to a set, and then back to an array.
const numbers = [1, 1, 20, 3, 3, 3, 9, 9];
const uniqueNumbers = [...new Set(numbers)]; // -> [1, 20, 3, 9]
Here’s how it works. new Set(numbers)
creates a set from a list of numbers. Creating a set automatically removes all duplicate values. The spread operator ...
converts any iterable to an array. This means [...new Set(numbers)]
converts the set right back to an array.
Source
https://medium.com/geekculture/20-javascript-snippets-to-code-like-a-pro-86f5fda5598e