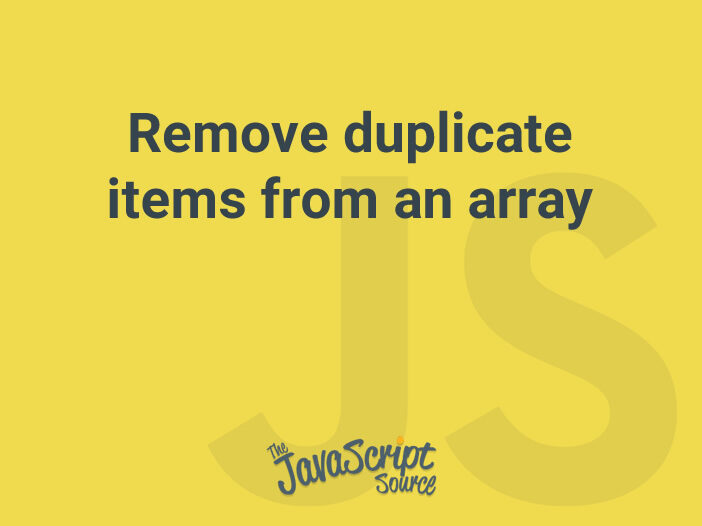
Let’s imagine you had an array of sandwiches
, and some of the items in it were listed more than once.
let sandwiches = ['turkey', 'ham', 'turkey', 'tuna', 'pb&j', 'ham', 'turkey', 'tuna'];
You want to get an updated list with the duplicates removed, a process called deduplication.
The new Set()
constructor creates an iterable collection of items, just like an array. But, each item can only be included once.
To remove any duplicates, we can pass our array into the new Set()
constructor, then convert the returned collection back into an array.
let deduped = Array.from(new Set(sandwiches));
Helper function:
/*!
* Remove duplicate items from an array
* (c) 2021 Chris Ferdinandi, MIT License, https://gomakethings.com
* @param {Array} arr The array
* @return {Array} A new array with duplicates removed
*/
function dedupe (arr) {
return Array.from(new Set(arr));
}