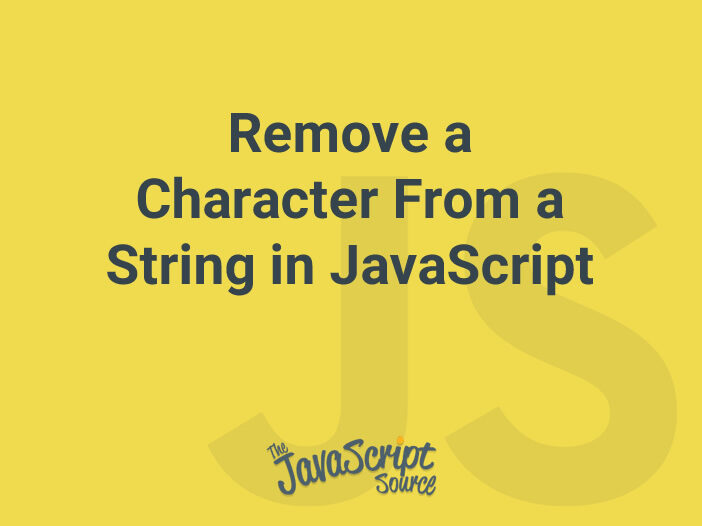
Here are a few different ways to remove a specific character from a string.
The replace
Method
The original purpose of this method is to replace a string with another string, but we can also use it to remove a character from a string by replacing it with an empty string. In the following example, the strWebsiteName
string variable contains the \n
character, and we want to remove it.
var strWebsiteName = "\ncode.tutsplus.com;
var strNewWebsiteName = strWebsiteName.replace("\n", "");
console.log(strNewWebsiteName);
//output: "code.tutsplus.com"
Note that the replace
method only replaces the first occurrence of a string in the source string. So if you want to remove all occurrences of a specific character from the source string, you need to use a regular expression in the replace
method.
The replace
Method With a Regular Expression
In the following example, we’ve used a regular expression in the first argument of the replace
method. It will remove all occurrences of the \n
character from the source string.
var strWebsiteName = "\ncode.tutsplus.com\n\n\n\n";
var strNewWebsiteName = strWebsiteName.replace(/(\n)/gm,"");
console.log(strNewWebsiteName);
//output: "code.tutsplus.com"
When you’re using a regular expression with the replace
method, you can use a callback function to process the replacement, as shown in the following example.
function removeCharCallback(match, replaceString, offset, string) {
return "";
}
var strWebsiteName = "\ncode.tutsplus.com\n\n\n\n";
var strNewWebsiteName = strWebsiteName.replace(/(\n)/gm, removeCharCallback);
console.log(strNewWebsiteName);
//output: "code.tutsplus.com"
The split
Method
Here’s how you can use the split
and join
methods to remove a character from a string in JavaScript.
var strWebsiteName = "\ncode.tutsplus.com\n\n\n\n";
var strNewWebsiteName = strWebsiteName.split("\n").join('');
console.log(strNewWebsiteName);
//output: "code.tutsplus.com"
Source
https://code.tutsplus.com/tutorials/how-to-remove-a-character-from-a-string-in-javascript–cms-37324