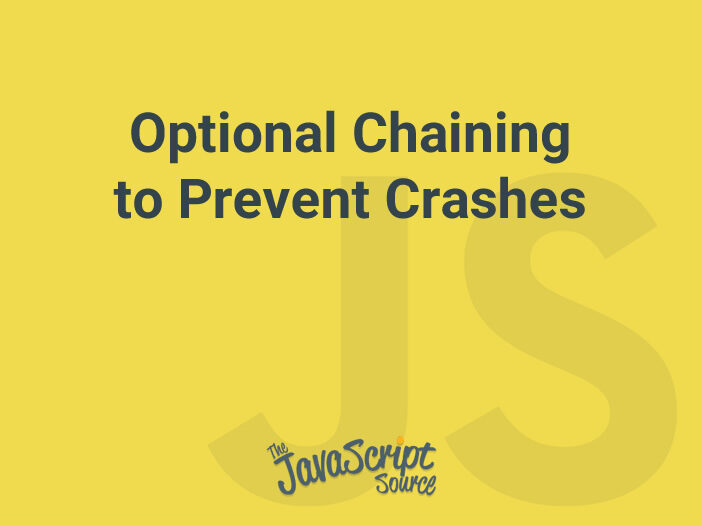
If you access an undefined property, an error is produced. This is where optional chaining comes to the rescue.
Using the optional chaining operator ?.
when the property is not defined, undefined
is returned instead of an error. This prevents your code from crashing.
For example:
const student = {
name: "Matt",
age: 27,
address: {
state: "New York"
},
};
// LONG FORM
console.log(student && student.address && student.address.ZIPCode); // Doesn't exist - Returns undefined
// SHORTHAND
console.log(student?.address?.ZIPCode); // Doesn't exist - Returns undefined
Source
https://medium.com/geekculture/20-javascript-snippets-to-code-like-a-pro-86f5fda5598e