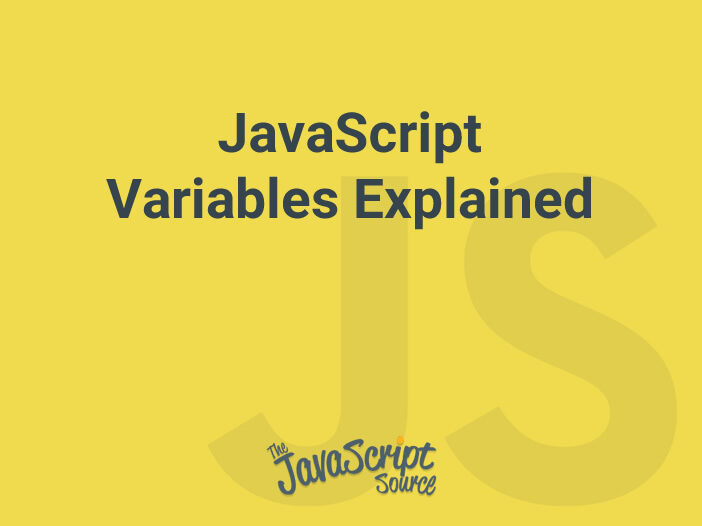
Variables are named values and can store any type of JavaScript value.
Here’s how to declare a variable:
var x = 100;
And here’s what’s happening in the example above:
- var is the keyword that tells JavaScript you’re declaring a variable.
- x is the name of that variable.
- = is the operator that tells JavaScript a value is coming up next.
- 100 is the value for the variable to store.
Using variables
After you declare a variable, you can reference it by name elsewhere in your code.
var x = 100;
x + 102;
// Output
202
Reassigning variables
You can give an existing variable a new value at any point after it’s declared.
var weather = "rainy";
weather = "sunny";
weather;
// Output
"sunny"