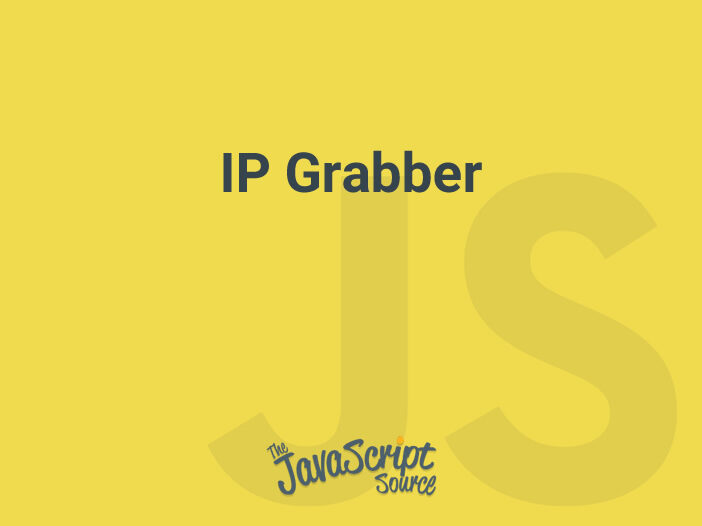
There are ways to get a users IP address in JavaScript, but it’s difficult. In Chrome specifically, they have implemented a stricter policy that prevents access to the RTCPeerConnection unless it is used over a secure origin (HTTPS or localhost).
The best way is to use a 3rd party API or write server-side code that you can call from a JavaScript function. Here’s an example using Ipify:
<SCRIPT LANGUAGE=”JavaScript”>
fetch('https://api.ipify.org?format=json')
.then(response => response.json())
.then(data => console.log(data.ip));
</script>
This will make a request to the ‘https://api.ipify.org?format=json‘ which is a free public API that returns the user’s IP address in JSON format.
Please note that this approach will reveal the user’s IP address to the server that you are making the request to, which may or may not be acceptable depending on your use case.
If you’re testing and want to know what your IP address is there are a variety of sites that help do this.