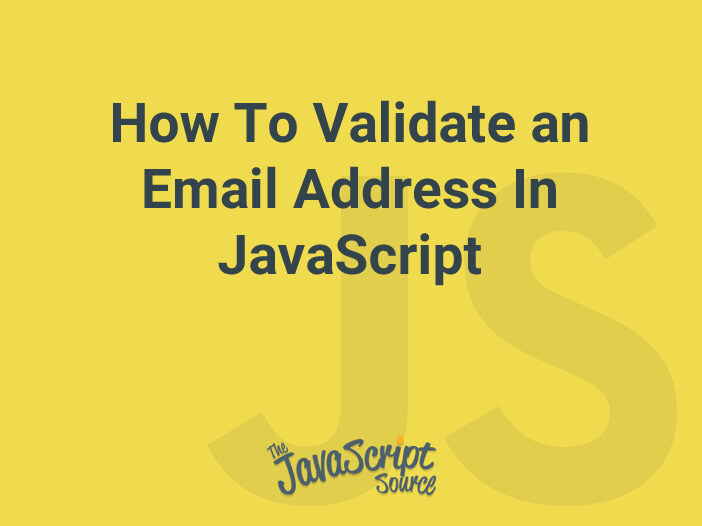
Email validation is a common task when working with forms in JavaScript. It involves checking that an email address entered by a user is in the correct format. In this article, we will discuss several methods for validating email addresses in JavaScript, including using regular expressions and built-in JavaScript methods.
Using a Regular Expression
One way to validate an email address is to use a regular expression. A regular expression is a sequence of characters that defines a search pattern. In this case, we can use a regular expression to check that the email address entered by the user matches the pattern of a valid email address.
Here’s an example of how to use a regular expression to validate an email address in JavaScript:
function validateEmail(email) {
var re = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
return re.test(String(email).toLowerCase());
}
console.log(validateEmail("[email protected]")); // true
console.log(validateEmail("[email protected]")); // false
In this example, the validateEmail
function takes an email address as its argument and uses the test
method of the regular expression to check if the email address matches the pattern. The test
method returns true
if the email address is valid and false
if it is not.
Using Built-in JavaScript Methods
Another way to validate an email address in JavaScript is to use built-in JavaScript methods such as indexOf
and lastIndexOf
.
Here’s an example of how to use these methods to validate an email address:
function validateEmail(email) {
var atpos = email.indexOf("@");
var dotpos = email.lastIndexOf(".");
if (atpos<1 || dotpos<atpos+2 || dotpos+2>=email.length) {
return false;
}
return true;
}
console.log(validateEmail("[email protected]")); // true
console.log(validateEmail("[email protected]")); // false
In this example, the validateEmail
function takes an email address as its argument and uses the indexOf
method to find the position of the @
symbol and the lastIndexOf
method to find the position of the last .
symbol. It then checks if the @
symbol is in the correct position and if the .
symbol is in the correct position. If the email address is valid, the function returns true
; otherwise, it returns false
.
Both of these methods will help you to validate an email address in JavaScript. You can choose the method that best suits your needs.
Note: Keep in mind that these are basic examples of email validation. There are other more complex validation techniques, such as checking for the existence of MX records for the domain