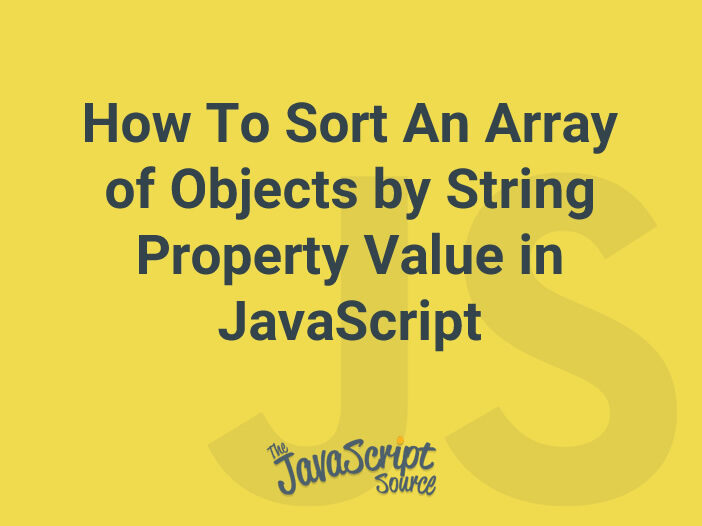
JavaScript provides several ways to sort an array of objects by a specific property value. One way is to use the sort()
method, which sorts the elements of an array in place and returns the sorted array. The sort()
method can take a comparison function as an argument, which specifies the sorting order.
Here’s an example of how to sort an array of objects by a string property value called “name”:
const data = [
{name: "John", age: 25},
{name: "Mary", age: 30},
{name: "Bob", age: 35},
{name: "Alice", age: 20}
];
data.sort(function(a, b) {
return a.name.localeCompare(b.name);
});
console.log(data);
This will output the following sorted array:
[
{name: "Alice", age: 20},
{name: "Bob", age: 35},
{name: "John", age: 25},
{name: "Mary", age: 30}
]
Note that we are using the localeCompare()
function inside the comparison function. It compares two strings in the current locale and returns a value indicating their sort order.
Another way is to use the sort()
method with the map()
method and the spread operator(...)
.
const data = [
{name: "John", age: 25},
{name: "Mary", age: 30},
{name: "Bob", age: 35},
{name: "Alice", age: 20}
];
const sortedData = [...data].sort((a, b) => a.name.localeCompare(b.name));
console.log(sortedData)
This will output the same as the above example.
It is also possible to sort the array in descending order by changing the comparison function to return the negation of the localeCompare()
function:
data.sort(function(a, b) {
return -a.name.localeCompare(b.name);
});
This will output the following sorted array in descending order:
[
{name: "Mary", age: 30},
{name: "John", age: 25},
{name: "Bob", age: 35},
{name: "Alice", age: 20}
]
It is important to note that the sort()
method modifies the original array, so if you want to keep the original array intact, you should create a copy of the array before sorting it.