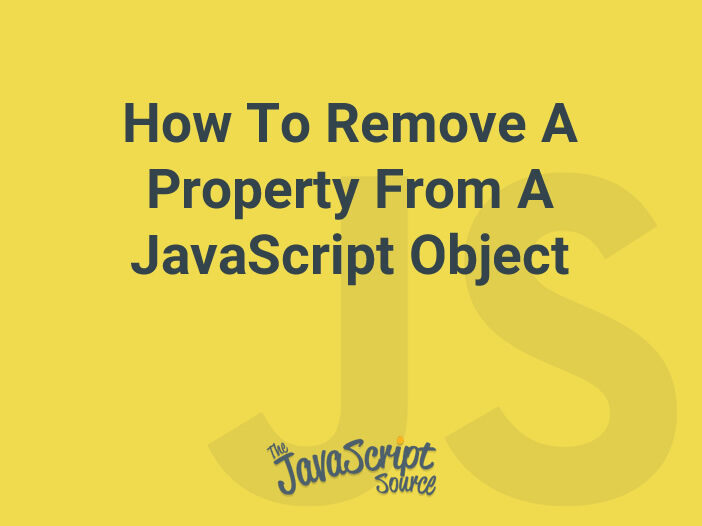
JavaScript provides several ways to remove a property from an object. One way is to use the delete
operator, which is used to delete a property from an object. Here is an example:
let obj = { name: 'John', age: 30 };
console.log(obj); // Output: { name: 'John', age: 30 }
delete obj.name;
console.log(obj); // Output: { age: 30 }
In this example, we first create an object with two properties: name
and age
. We then use the delete
operator to remove the name
property from the object. After the delete operator is used, the object no longer has the name
property and its value is undefined.
Another way to remove a property from an object is to use the Object.defineProperty()
method. This method can be used to change the property descriptor of an existing property, which includes the ability to make the property non-configurable and therefore unable to be deleted. Here’s an example:
let obj = { name: 'John', age: 30 };
console.log(obj); // Output: { name: 'John', age: 30 }
Object.defineProperty(obj, 'name', {
configurable: false
});
delete obj.name;
console.log(obj); // Output: { name: 'John', age: 30 }
In this example, we first create an object with two properties: name
and age
. We then use the Object.defineProperty()
method to make the name
property non-configurable. After that, we try to delete the name
property but it’s still there.
Lastly, we can use the Object.assign()
method to remove a property from an object. The Object.assign()
method creates a new object, which is a copy of the original object without the specified property. Here’s an example:
let obj = { name: 'John', age: 30 };
console.log(obj); // Output: { name: 'John', age: 30 }
let newObj = Object.assign({}, obj);
delete newObj.name;
console.log(newObj); // Output: { age: 30 }
In this example, we first create an object with two properties: name
and age
. We then use the Object.assign()
method to create a new object, which is a copy of the original object. We then delete the name
property from the new object.