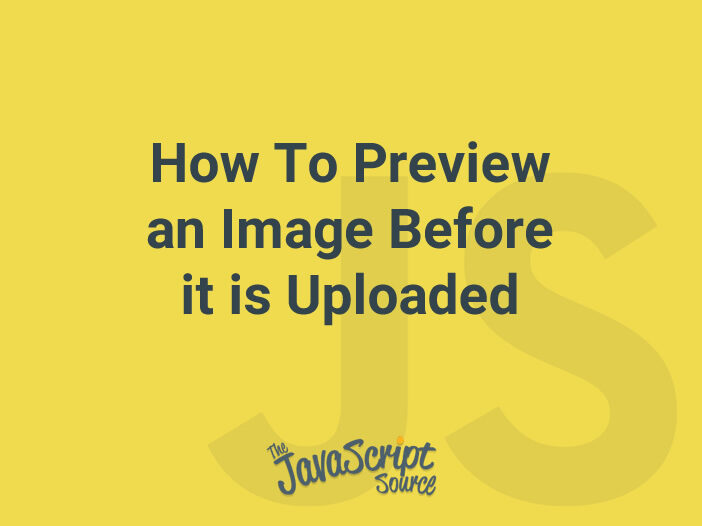
Previewing an image before it is uploaded is a useful feature that allows users to verify the image they have selected before submitting it to the server. This can be achieved using JavaScript and the HTML5 File API.
First, you will need to create an HTML form that includes a file input field and a preview area for the image. Here is an example:
<form>
<input type="file" id="fileInput" onchange="previewImage()" />
<div id="preview"></div>
</form>
The onchange
event is used to call the previewImage()
function when a file is selected. The id
attribute is used to reference the input field and the preview area in JavaScript.
Next, you will need to create the previewImage()
function. This function will get the selected file, create an object URL for the file, and set the src
attribute of an img
element to the object URL. Here is an example:
function previewImage() {
var fileInput = document.getElementById('fileInput');
var file = fileInput.files[0];
var objectUrl = URL.createObjectURL(file);
var preview = document.getElementById('preview');
preview.innerHTML = '<img src="' + objectUrl + '" />';
}
In this example, the fileInput
variable is used to reference the file input field, the file
variable is used to reference the selected file, the objectUrl
variable is used to create an object URL for the file, and the preview
variable is used to reference the preview area.
Finally, you can style the preview area to make it look more attractive.
#preview {
width: 300px;
height: 300px;
overflow: hidden;
}
#preview img {
width: 100%;
}
Note that when you no longer need the object URL, you should revoke it by calling URL.revokeObjectURL(objectUrl)
to free up memory. You can also add some validation to your code to check if the file selected is an image file or not, using the file.type
property. This can be done inside the previewImage()
function before creating the object URL.