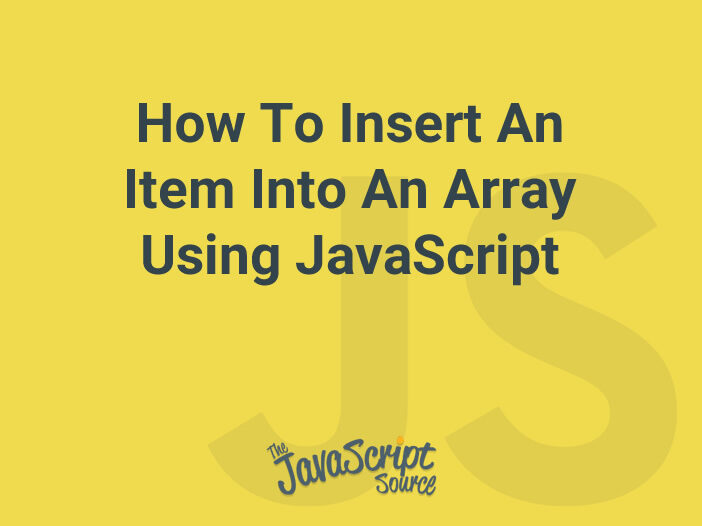
Inserting an item into an array in JavaScript is a simple task that can be accomplished using a few different methods. In this article, we will explore three different ways to insert an item into an array in JavaScript, including using the push()
method, the splice()
method, and the concat()
method.
The push()
method is one of the most commonly used methods for inserting an item into an array. This method adds one or more items to the end of an array and returns the new length of the array. The syntax for using the push()
method is as follows:
array.push(item1, item2, ...);
For example, if we have an array called colors
and we want to insert the color “purple” at the end of the array, we would use the following code:
colors.push("purple");
The splice()
method is another method that can be used to insert an item into an array. This method allows you to add or remove items from an array at a specific index. The syntax for using the splice()
method is as follows:
array.splice(index, 0, item);
For example, if we have an array called numbers
and we want to insert the number 4 at the second index of the array, we would use the following code:
numbers.splice(1, 0, 4);
The concat()
method is a third method that can be used to insert an item into an array. This method combines two or more arrays into a new array. The syntax for using the concat()
method is as follows:
newArray = array1.concat(array2, array3, ...);
For example, if we have an array called fruits
and we want to insert the array ["bananas", "oranges"]
at the end of the fruits
array, we would use the following code:
fruits = fruits.concat(["bananas", "oranges"]);