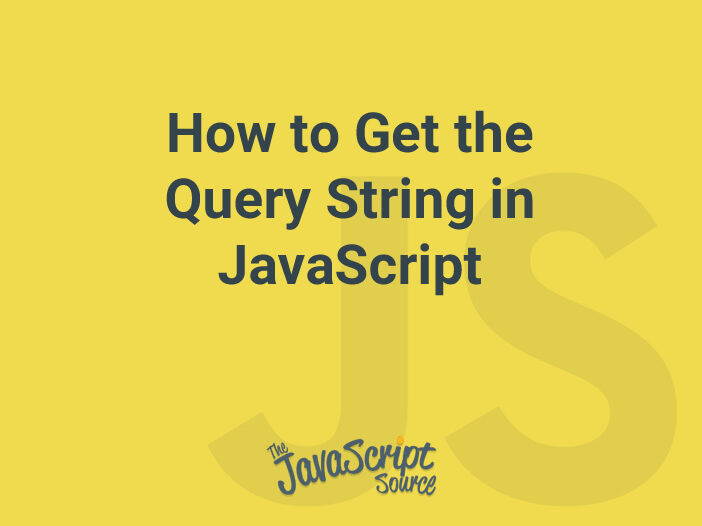
When you’re working with JavaScript, sometimes you need to access query string parameters in your script.
Let’s go through the following JavaScript example.
function getQueryStringValues(key) {
var arrParamValues = [];
var url = window.location.href.slice(window.location.href.indexOf('?') + 1).split('&');
for (var i = 0; i < url.length; i++) {
var arrParamInfo = url[i].split('=');
if (arrParamInfo[0] == key || arrParamInfo[0] == key+'[]') {
arrParamValues.push(decodeURIComponent(urlparam[1]));
}
}
return (arrParamValues.length > 0 ? (arrParamValues.length == 1 ? arrParamValues[0] : arrParamValues) : null);
}
// index.php?keyword=FooBar&hobbies[]=sports&hobbies[]=Reading
console.log(getQueryStringValues('keyword')); // "FooBar"
console.log(getQueryStringValues('hobbies')); // Array [ "sports", "Reading" ]
console.log(getQueryStringValues('keyNotExits')); // null
We’ve made the getQueryStringValues
function, which you can use to get the value of the query string parameter available in the URL.
Let’s go through the function to see how it works.
The following snippet is one of the most important snippets in the function.
var url = window.location.href.slice(window.location.href.indexOf('?') + 1).split('&');
Firstly, we’ve used the indexOf
method to find the position of the ?
character in the URL. Next, we’ve used the slice
method to extract the query string part in the URL. Finally, we’ve used the split
method to split the query string by the &
character. Thus, the url
variable is initialized with an array of query string parameters.
Next, we loop through all the elements of the url
array. In the loop, we use the split
method to split the array value by the =
character. And with that, the arrParamInfo
variable is initialized with an array, where the array key is the parameter name and the array value is the parameter value. You can see that in the following snippet.
var arrParamInfo = url[i].split('=');
Next, we compare it with the argument which is passed in the function. If it matches the incoming argument, we’ll push the parameter value into the arrParamValues
array. As you can see, we’ve covered both single and array parameters as well.
if (arrParamInfo[0] == key || arrParamInfo[0] == key+'[]') {
arrParamValues.push(decodeURIComponent(urlparam[1]));
}
Finally, if the arrParamValues
variable contains values, we’ll return it, otherwise null
is returned.
return (arrParamValues.length > 0 ? (arrParamValues.length == 1 ? arrParamValues[0] : arrParamValues) : null);
You can go ahead and test the getQueryStringValues
function with different values.
As shown in the above example, we’ve called it with different values and logged the output with the console.log
function. It’s important to note that if the parameter which you’ve passed in the getQueryStringValues
function exists as an array in the query string, you would get an array in response, and it would return all the values of that parameter.
Source
https://code.tutsplus.com/tutorials/how-to-get-the-query-string-in-javascript–cms-37024