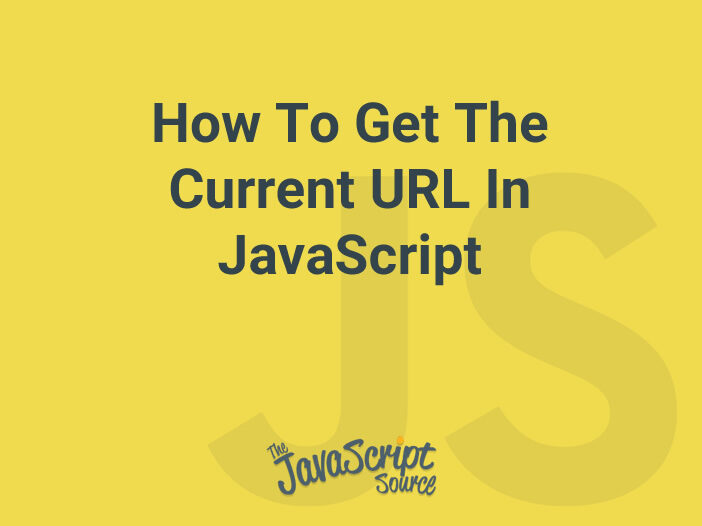
JavaScript provides multiple ways to get the current URL of a webpage. In this article, we will discuss the most common methods to achieve this.
Method 1: Using the window.location
object
The window.location
object is a property of the window
object and contains information about the current URL. The href
property of this object returns the entire URL of the current page, including the protocol (http or https), domain, and path. Here’s an example:
console.log(window.location.href);
Method 2: Using the document.URL
property
The document.URL
property is another way to get the current URL of a webpage. It returns the entire URL, including the protocol, domain, and path. Here’s an example:
console.log(document.URL);
Method 3: Using the document.location.href
property
This method also provides the entire URL, including the protocol, domain, and path. The document.location
object is a property of the document
object and contains information about the current URL. The href
property of this object returns the entire URL.
console.log(document.location.href);
All of the above methods will give you the current URL of the webpage. You can choose any of these methods based on your requirement and use it in your JavaScript code.
Note: It is worth mentioning that you can also access other parts of the url like for example
console.log(window.location.hostname) // will return the hostname (www.example.com)
console.log(window.location.pathname) // will return the path '/about'
console.log(window.location.protocol) // will return the protocol 'http:'
and many other properties.