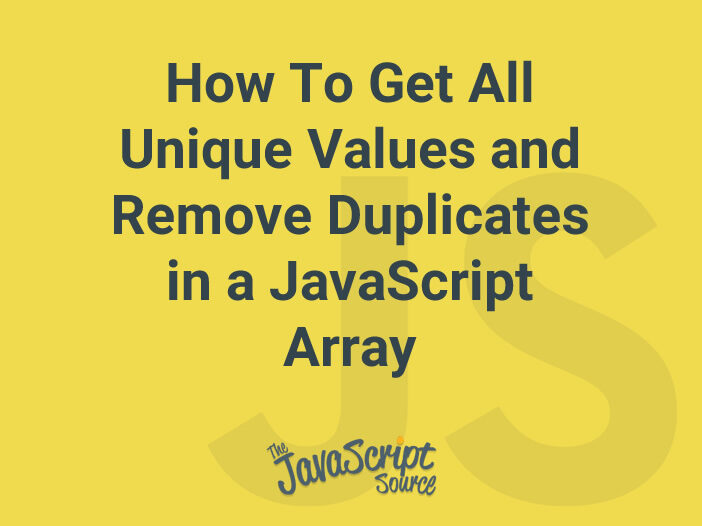
JavaScript arrays are a powerful and versatile data structure for storing collections of items. However, sometimes arrays may contain duplicate values, which can cause issues when working with the data. In this article, we will explain how to get all unique values and remove duplicates in a JavaScript array.
To get all unique values in an array, you can use the Set()
constructor. The Set()
constructor creates a new Set object, which can only contain unique values. To get the unique values of an array, you can pass the array to the Set()
constructor, and then convert it back to an array using the Array.from()
method.
Here is an example of how to get all unique values in an array:
let numbers = [1, 2, 3, 3, 4, 4, 5, 5];
let uniqueNumbers = Array.from(new Set(numbers));
console.log(uniqueNumbers); // Output: [1, 2, 3, 4, 5]
In this example, the numbers
array contains some duplicate values. By passing this array to the Set()
constructor, we are able to remove the duplicates and create a new uniqueNumbers
array that only contains unique values.
To remove duplicates from an array, you can use the filter()
method. The filter()
method takes a callback function as an argument, which is called for each element in the array. The callback function should return true
for elements that should be included in the new array, and false
for elements that should be excluded. To remove duplicates, you can use the indexOf()
method to check if the current element is the first occurrence of that value in the array.
Here is an example of how to remove duplicates from an array:
let numbers = [1, 2, 3, 3, 4, 4, 5, 5];
let noDuplicates = numbers.filter((item, index) => numbers.indexOf(item) === index);
console.log(noDuplicates); // Output: [1, 2, 3, 4, 5]
In this example, the filter()
method is called for each element in the numbers
array. The callback function checks if the current element is the first occurrence of that value in the array by comparing the index of the current element to the index of the first occurrence of that value using the indexOf()
method. If they are equal, the current element is included in the new noDuplicates
array, otherwise it is excluded.