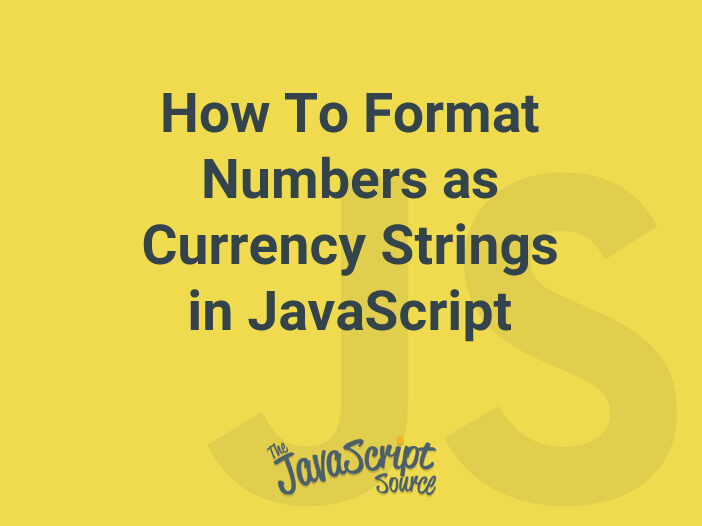
JavaScript provides several ways to format numbers as currency strings. One of the most commonly used methods is the toLocaleString()
method, which allows you to specify the locale and currency to be used when formatting the number.
Here is an example of how to use the toLocaleString()
method to format a number as a currency string in the US locale:
let number = 123456.789;
let options = { style: 'currency', currency: 'USD' };
let currencyString = number.toLocaleString('en-US', options);
console.log(currencyString); // "$123,456.79"
In this example, the number
variable holds the number to be formatted, and the options
variable holds an object with the desired formatting options. The toLocaleString()
method is called with the locale (‘en-US’) and the options as arguments, and the result is stored in the currencyString
variable.
Another way to format a number as a currency string is using Intl.NumberFormat()
.
let number = 123456.789;
let currency = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD'
});
console.log(currency.format(number)); // "$123,456.79"
In this example, the number
variable holds the number to be formatted, and the Intl.NumberFormat()
method is called with the locale (‘en-US’) and the options as arguments, and the result is stored in the currency
variable.
Note that both the examples above will format the number with the currency symbol and the appropriate number of decimal places for the specified currency. The toLocaleString()
method and Intl.NumberFormat()
will also take care of any necessary grouping of digits (e.g. adding commas as thousands separators).
You can also use template literals to format the number as currency.
let number = 123456.789;
let currencyString = `$ ${number.toFixed(2)}`;
console.log(currencyString); // "$ 123456.79"
In this example, the number
variable holds the number to be formatted, and the toFixed(2)
method is called with the number of decimal places as an argument. The resulting value is then concatenated with the currency symbol “$” using template literals.