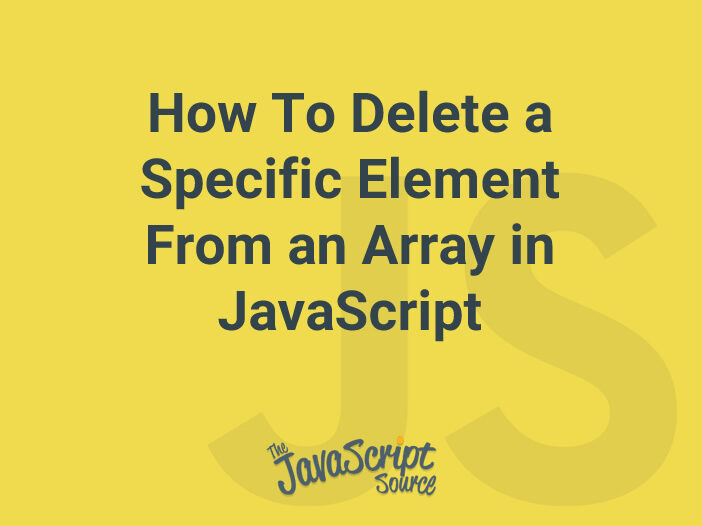
In JavaScript, there are several ways to remove a specific element from an array. The most common methods include using the splice()
method, the filter()
method, and the indexOf()
method.
The splice()
method is used to remove elements from an array by specifying the starting index and the number of elements to remove. The syntax for the splice()
method is as follows:
array.splice(index, numberOfElements)
For example, if you have an array called “fruits” and you want to remove the element “banana” from the array, you can use the following code:
let fruits = ["apple", "banana", "orange"];
let index = fruits.indexOf("banana");
fruits.splice(index, 1);
console.log(fruits); // ["apple", "orange"]
In this example, the indexOf()
method is used to find the index of the element “banana” in the array. The splice()
method is then used to remove the element at that index, with 1 specifying the number of elements to remove.
Another method to remove a specific element from an array is the filter()
method. This method creates a new array with all elements that pass the test implemented by the provided function. Here is an example of how to use the filter()
method to remove the element “banana” from the array “fruits”:
let fruits = ["apple", "banana", "orange"];
fruits = fruits.filter(fruit => fruit !== "banana");
console.log(fruits); // ["apple", "orange"]
In this example, the filter()
method creates a new array with all elements that do not match the element “banana”. The original array “fruits” is then reassigned to the new array, effectively removing the element “banana”.
Lastly, you can use the indexOf
method to find the index of an element in an array and use the delete operator to remove the element. The delete operator will set the element to undefined instead of removing it.
let fruits = ["apple", "banana", "orange"];
let index = fruits.indexOf("banana");
delete fruits[index];
console.log(fruits); // ["apple", undefined, "orange"]
It depends on your specific use case which method to use.