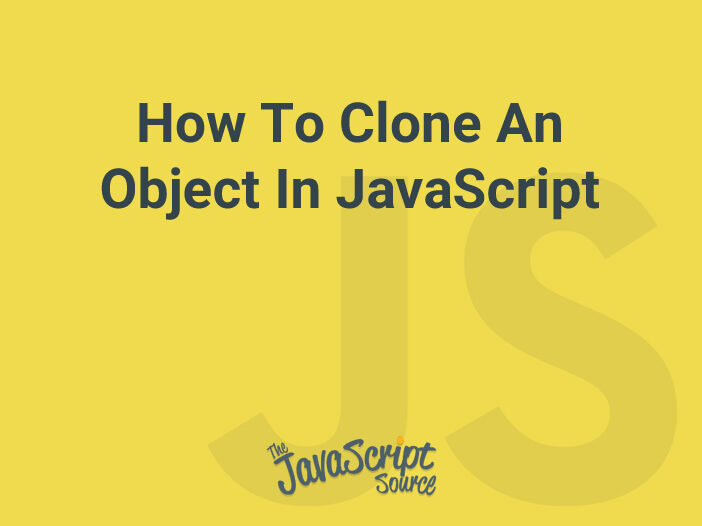
JavaScript provides several ways to clone an object, each with its own set of advantages and limitations. In this article, we will discuss three common methods for cloning objects in JavaScript:
The Spread Operator
This method uses the spread operator (...
) to create a new object with the same properties as the original. It is a shallow copy, which means that if the original object contains any nested objects, the copy will still reference the same nested objects as the original.
let original = {a: 1, b: 2};
let copy = {...original};
console.log(copy); // {a: 1, b: 2}
Object.assign()
This method uses the Object.assign()
function to create a new object and copy the properties of the original object to it. Like the spread operator, this method also creates a shallow copy.
let original = {a: 1, b: 2};
let copy = Object.assign({}, original);
console.log(copy); // {a: 1, b: 2}
JSON.parse(JSON.stringify(obj))
This method uses the JSON.stringify()
method to convert the original object to a JSON string, and then uses the JSON.parse()
method to create a new object from the JSON string. This method creates a deep copy of the original object, meaning that any nested objects will be copied as well and the copy will not reference the same nested objects as the original.
let original = {a: 1, b: 2, c: {d:3}};
let copy = JSON.parse(JSON.stringify(original));
console.log(copy); // {a: 1, b: 2, c: {d:3}}
It’s important to note that all three methods have different performance characteristics, and the method you choose will depend on the size and complexity of the object you’re trying to clone, as well as the requirements of your project. The spread operator and Object.assign()
are generally faster than JSON.parse(JSON.stringify())
, but they only create shallow copies, so if you need a deep copy, you’ll need to use the JSON.parse(JSON.stringify())
method.