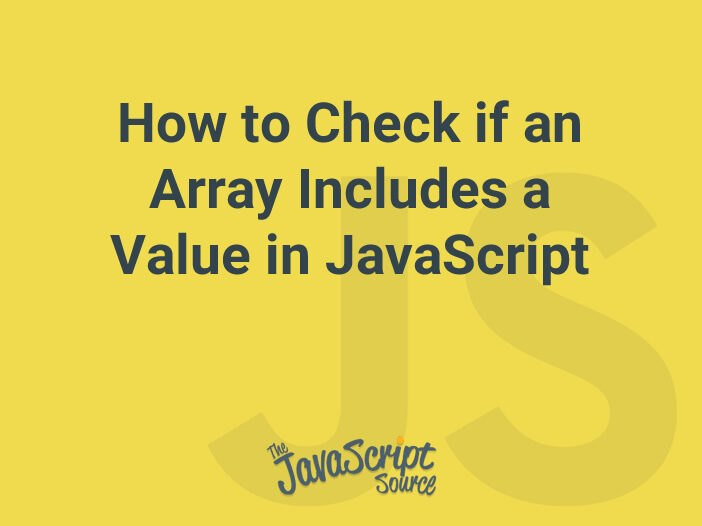
JavaScript offers several ways to check if an array includes a specific value. In this article, we will explore some of the most common methods for checking for the presence of a value in an array.
The first method we will look at is the indexOf()
method. This method returns the index of the first occurrence of the specified value in the array, or -1 if the value is not found.
let fruits = ["apple", "banana", "orange"];
let index = fruits.indexOf("banana");
console.log(index); // 1
In the example above, the indexOf()
method returns the index of “banana” in the fruits array, which is 1. If we were to check for a value that does not exist in the array, such as “grape”, the method would return -1.
let fruits = ["apple", "banana", "orange"];
let index = fruits.indexOf("grape");
console.log(index); // -1
Another method for checking if an array includes a value is the includes()
method. This method returns a boolean value indicating whether the specified value is present in the array.
let fruits = ["apple", "banana", "orange"];
let hasBanana = fruits.includes("banana");
console.log(hasBanana); // true
In the example above, the includes()
method returns true
because “banana” is present in the fruits array. If we were to check for a value that does not exist in the array, such as “grape”, the method would return false
.
let fruits = ["apple", "banana", "orange"];
let hasGrape = fruits.includes("grape");
console.log(hasGrape); // false
We can also use the find()
method to check if an array includes a value. This method returns the first element in the array that satisfies the provided testing function.
let fruits = ["apple", "banana", "orange"];
let hasBanana = fruits.find(fruit => fruit === "banana");
console.log(hasBanana); // "banana"
In the example above, the find()
method returns “banana” because it is the first element in the fruits array that satisfies the provided testing function. If we were to check for a value that does not exist in the array, such as “grape”, the method would return undefined
.
let fruits = ["apple", "banana", "orange"];
let hasGrape = fruits.find(fruit => fruit === "grape");
console.log(hasGrape); // undefined