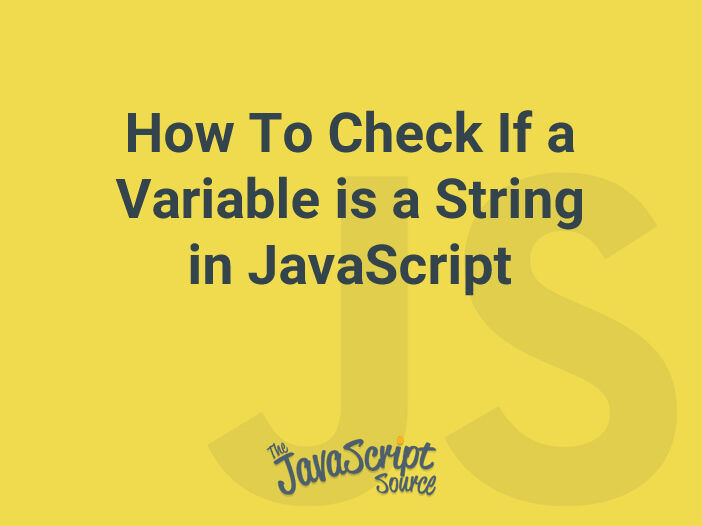
JavaScript provides several ways to check if a variable is a string. One of the most common methods is to use the typeof
operator, which returns the type of a variable. For example, the following code checks if a variable named “myVar” is a string:
if (typeof myVar === "string") {
console.log("myVar is a string");
} else {
console.log("myVar is not a string");
}
Another way to check if a variable is a string is to use the instanceof
operator, which returns true if an object is an instance of a particular constructor. For example, the following code checks if a variable named “myVar” is an instance of the String constructor:
if (myVar instanceof String) {
console.log("myVar is a string");
} else {
console.log("myVar is not a string");
}
Another way to check if a variable is a string is to use the Object.prototype.toString.call()
method. This method returns a string representing the object’s constructor. For example, the following code checks if a variable named “myVar” is a string:
if (Object.prototype.toString.call(myVar) === "[object String]") {
console.log("myVar is a string");
} else {
console.log("myVar is not a string");
}
Lastly, we can use the constructor
property
if(myVar.constructor === String) {
console.log("myVar is a string");
} else {
console.log("myVar is not a string");
}