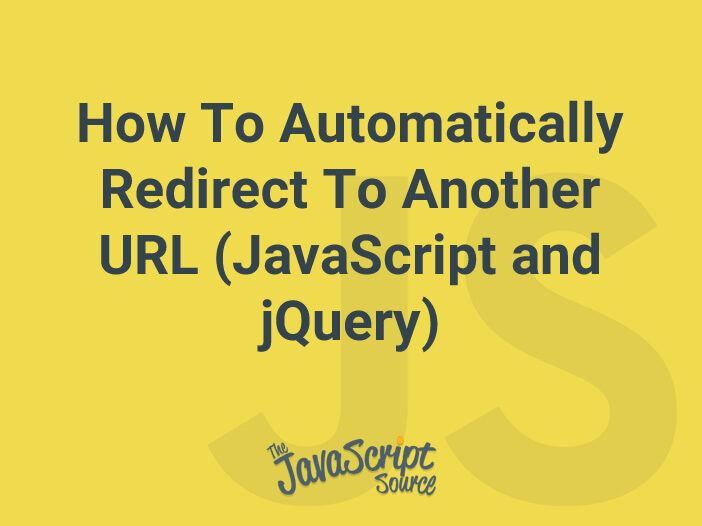
JavaScript and jQuery are both popular JavaScript libraries that can be used to redirect a page to another URL on page load. In this article, we will explain how to accomplish this task using both JavaScript and jQuery, with code examples for each method.
Using JavaScript:
The simplest way to redirect a page to another URL on page load using JavaScript is to use the location.href
property. This property can be set to any valid URL, and the browser will automatically redirect the page to that URL. Here is an example of how to use this method:
window.onload = function() {
location.href = "https://www.example.com";
}
In this example, the onload
event is used to trigger the redirect function, which sets the location.href
property to the desired URL. This code should be placed in the head of your HTML document, or in an external JavaScript file that is included in your HTML document.
Using jQuery:
Another way to redirect a page to another URL on page load using jQuery is to use the ready
function, which is triggered when the page has finished loading. Here is an example of how to use this method:
$(document).ready(function() {
window.location.href = "https://www.example.com";
});
In this example, the ready
function is used to trigger the redirect function, which sets the location.href
property to the desired URL. This code should be placed in the head of your HTML document, or in an external JavaScript file that is included in your HTML document.
Note: you need to include jQuery library before using it.