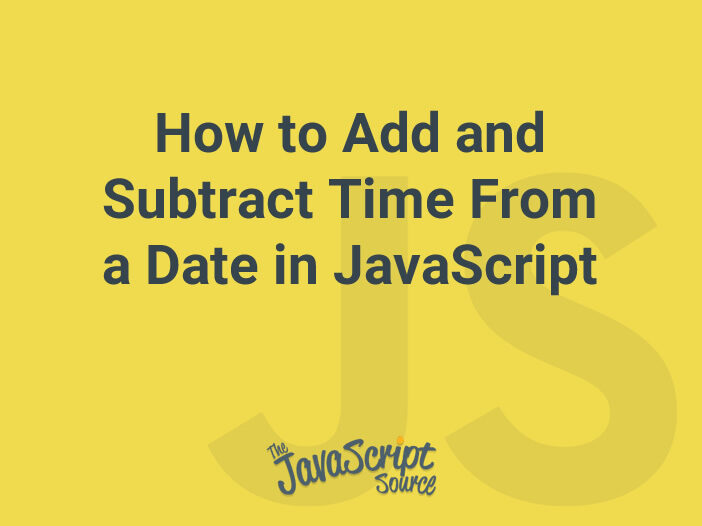
How to Add Time to a JavaScript Date With Vanilla JavaScript
In JavaScript, the getTime()
function returns the number of milliseconds since the Unix epoch time. That’s just a computer time convention that counts the number of seconds (milliseconds in JavaScript) since midnight UTC on January 1st, 1970.
Let’s try to understand how you can use the getTime()
function to add time to a Date
object in JavaScript. In the following example, we’ll add one hour to the existing Date
object.
var currentDateObj = new Date();
var numberOfMlSeconds = currentDateObj.getTime();
var addMlSeconds = 60 * 60 * 1000;
var newDateObj = new Date(numberOfMlSeconds + addMlSeconds);
// Results
currentDateObj: Sat Jul 24 2021 00:19:27 GMT-0300 (Atlantic Daylight Time)
newDateObj: Sat Jul 24 2021 01:19:27 GMT-0300 (Atlantic Daylight Time)
Firstly, we’ve initialized the currentDateObj
variable with the current date, and it’s a Date
object. Next, we’ve used the getTime()
function to get the number of milliseconds from the currentDateObj
object.
Next, we’ve calculated the number of milliseconds in an hour. Basically, we’ve just multiplied the number of minutes in an hour (60) by the number of seconds in a minute (60) by the number of milliseconds in a second (1000) to get the number of milliseconds in an hour.
As you might already know, you can initialize a Date
object by providing the number of milliseconds as the first argument, and this would initialize a date object in reference to it. Thus, we’ve added numberOfMlSeconds
and addMlSeconds
to get the total number of milliseconds, and we’ve used it to initialize a new date object. And the end result is the newDateObj
object, which should show a date ahead by one hour compared to the currentDateObj
object.
In fact, you can go ahead and make a reusable function, as shown in the following snippet.
function addHoursToDate(objDate, intHours) {
var numberOfMlSeconds = objDate.getTime();
var addMlSeconds = (intHours * 60) * 60 * 1000;
var newDateObj = new Date(numberOfMlSeconds + addMlSeconds);
return newDateObj;
}
Here’s how you can call it to get, for example, a date exactly one day in the future.
addHoursToDate(Date.now(), 24)
// Result
Sat Jul 25 2021 00:14:01 GMT-0300 (Atlantic Daylight Time)
So that’s how you can use the getTime()
function to add time to a Date object in JavaScript. You can also use the above function to add a number of days to an existing Date object; you just need to convert days to hours before calling the above function.
How to Subtract Time From a JavaScript Date With Vanilla JavaScript
As we’ve already discussed in the previous section, you can use the getTime()
function to get the number of milliseconds from a Date
object. And thus, the logic of subtracting time from a Date
object is very similar to the add operation, except that we need to subtract milliseconds instead of adding them.
Let’s go through the following example to see how it works.
function subtractTimeFromDate(objDate, intHours) {
var numberOfMlSeconds = objDate.getTime();
var addMlSeconds = (intHours * 60) * 60 * 1000;
var newDateObj = new Date(numberOfMlSeconds - addMlSeconds);
return newDateObj;
}
As you can see, it’s almost identical to the add operation. The only difference is that we’re subtracting addMlSeconds
from numberOfMlSeconds
instead of adding.
Source
https://code.tutsplus.com/tutorials/how-to-add-and-subtract-time-from-a-date-in-javascript–cms-37207