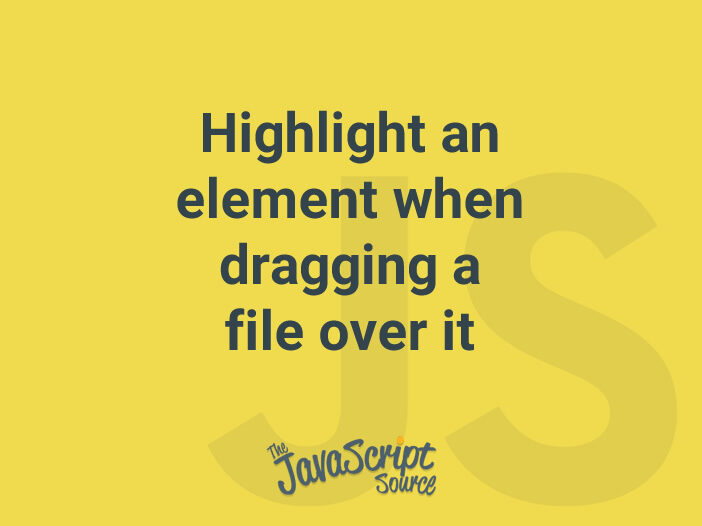
Assume that we have a droppable element as below:
<div id="droppable">...</div>
We will highlight the element when user drags a file over it. For example, the element will have a dashed border which can be simulated by a CSS class:
.dragging {
border: 4px dashed #ccc;
}
The dragging
class will be added to the element when user drags file and moves it over the element:
// Query the element
const ele = document.getElementById('droppable');
ele.addEventListener('dragenter', function (e) {
e.preventDefault();
e.target.classList.add('dragging');
});
In similar events, the class is removed from the element when user moves the file out of the element, or drops it:
ele.addEventListener('dragover', function (e) {
e.preventDefault();
});
ele.addEventListener('dragleave', function (e) {
e.preventDefault();
e.target.classList.remove('dragging');
});
ele.addEventListener('drop', function (e) {
e.preventDefault();
e.target.classList.remove('dragging');
});
The last thing, e.preventDefault()
is used in the handlers to prevent the browser from executing the default action.
Source
https://htmldom.dev/highlight-an-element-when-dragging-a-file-over-it/