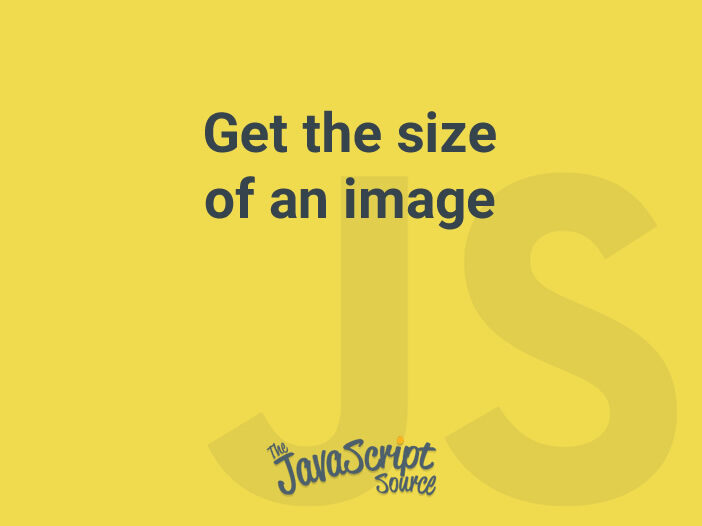
These snippets will get the height and width of an image, one when it is loaded and another when the image is not loaded yet.
Image is already loaded
const image = document.querySelector(...);
// Get the original size
const naturalWidth = image.naturalWidth;
const naturalHeight = image.naturalHeight;
// Get the scaled size
const width = image.width;
const height = image.height;
Image is not loaded yet
Listen on the load
event to calculate the size of image which can be loaded via a given URL:
const image = document.createElement('img');
image.addEventListener('load', function(e) {
// Get the size
const width = e.target.width;
const height = e.target.height;
});
// Set the source
image.src = '/path/to/image.png';
We can use a Promise
to turn the snippet to a reusable function:
const calculateSize = function(url) {
return new Promise(function(resolve, reject) {
const image = document.createElement('img');
image.addEventListener('load', function(e) {
resolve({
width: e.target.width,
height: e.target.height,
});
});
image.addEventListener('error', function() {
reject();
});
image.src = url;
});
};
calculateSize('/path/to/image.png').then(function(data) {
const width = data.width;
const height = data.height;
});