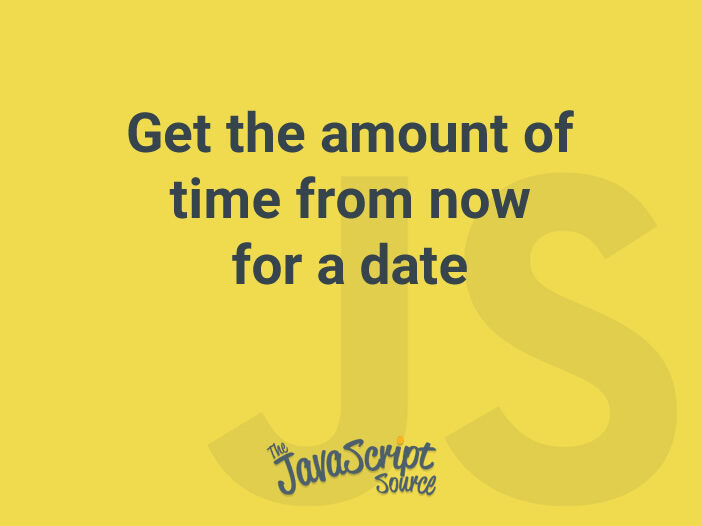
Here is a vanilla JS alternative to the moment.js timeFromNow()
method. The moment().timeFromNow()
method returns a formatted string with how long ago something happened.
You pass in a date from the past, and it returns things like a few seconds ago
or 2 years ago
.
// 4 years ago
moment([2007, 0, 29]).fromNow();
Instead of returning a string, our helper function will return an object with the difference in time, the time units, and whether the time is in the past or future.
/*!
* Get the amount of time from now for a date
* (c) 2021 Chris Ferdinandi, MIT License, https://gomakethings.com
* @param {String|Date} time The date to get the time from now for
* @return {Object} The time from now data
*/
function timeFromNow (time) {
// Get timestamps
let unixTime = new Date(time).getTime();
if (!unixTime) return;
let now = new Date().getTime();
// Calculate difference
let difference = (unixTime / 1000) - (now / 1000);
// Setup return object
let tfn = {};
// Check if time is in the past, present, or future
tfn.when = 'now';
if (difference > 0) {
tfn.when = 'future';
} else if (difference < -1) {
tfn.when = 'past';
}
// Convert difference to absolute
difference = Math.abs(difference);
// Calculate time unit
if (difference / (60 * 60 * 24 * 365) > 1) {
// Years
tfn.unitOfTime = 'years';
tfn.time = Math.floor(difference / (60 * 60 * 24 * 365));
} else if (difference / (60 * 60 * 24 * 45) > 1) {
// Months
tfn.unitOfTime = 'months';
tfn.time = Math.floor(difference / (60 * 60 * 24 * 45));
} else if (difference / (60 * 60 * 24) > 1) {
// Days
tfn.unitOfTime = 'days';
tfn.time = Math.floor(difference / (60 * 60 * 24));
} else if (difference / (60 * 60) > 1) {
// Hours
tfn.unitOfTime = 'hours';
tfn.time = Math.floor(difference / (60 * 60));
} else {
// Seconds
tfn.unitOfTime = 'seconds';
tfn.time = Math.floor(difference);
}
// Return time from now data
return tfn;
}