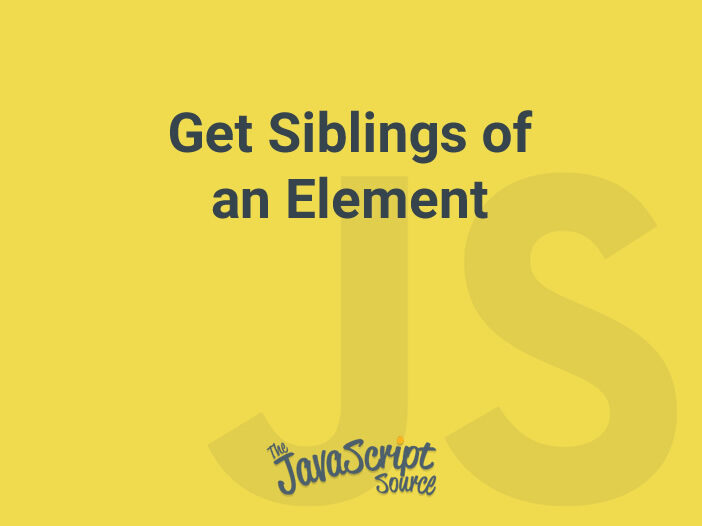
This snippet gets the next sibling and previous sibling of an element, using the element.nextSibling
and element.previousSibling
properties.
const previous = element.previousSibling;
const next = element.nextSibling;
The following helper function gets all the following sibling of an element:
const getNextSiblings = (e) => {
let siblings = [];
while (e = e.nextSibling) {
siblings.push(e);
}
return siblings;
}
And the following helper function gets all the previous siblings of an element:
const getPreviousSiblings = (e) => {
let siblings = [];
while (e = e.previousSibling) {
siblings.push(e);
}
return siblings;
}
The following helper function gets all siblings of an element:
const getSiblings = (e) => {
let siblings = [];
e = e.parentNode.firstChild;
do {
siblings.push(e);
} while (e = e.nextSibling);
return siblings;
}
You can add a filter function to the helper function as shown in the following example:
const getSiblings = (e, filter) => {
let siblings = [];
e = e.parentNode.firstChild;
do {
if (!filter || filter(e)) {
siblings.push(e);
}
} while (e = e.nextSibling);
return siblings;
}
The following example uses the getSiblings()
helper function to get all the siblings of an anchor element, which are also anchor elements:
const e = document.querySelector('a.first');
let links = getSiblings(el, (e) => {
e.nodeName.toLowerCase() === 'a';
});
Source
https://www.javascripttutorial.net/dom/traversing/get-siblings-of-an-element/