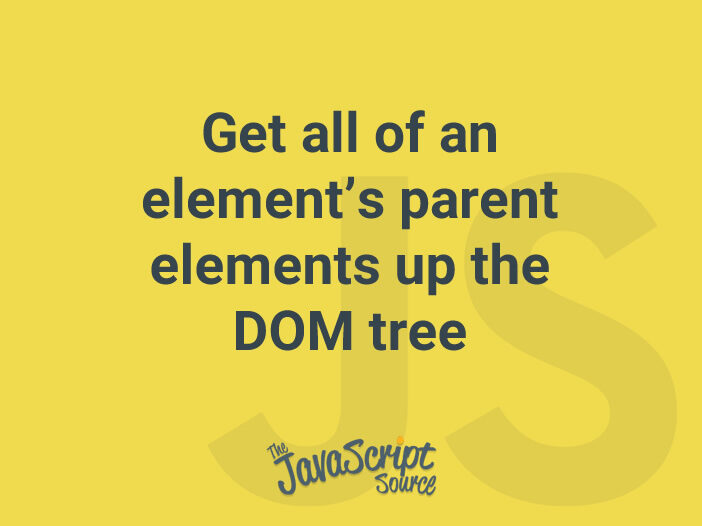
Let’s look at how to get all parent elements of a specific element.
/*!
* Get all of an element's parent elements up the DOM tree
* (c) 2021 Chris Ferdinandi, MIT License, https://gomakethings.com
* @param {Node} elem The element
* @param {Function} callback The test condition
* @return {Array} The parent elements
*/
function getParents (elem, callback) {
// Setup variables
let parents = [];
let parent = elem.parentNode;
let index = 0;
// Make sure callback is valid
if (typeof callback !== 'function') {
callback = null;
}
// Get matching parent elements
while (parent && parent !== document) {
// If using a selector, add matching parents to array
// Otherwise, add all parents
if (callback) {
if (callback(parent, index, elem)) {
parents.push(parent);
}
} else {
parents.push(parent);
}
// Jump to the next parent node
index++;
parent = parent.parentNode;
}
return parents;
}