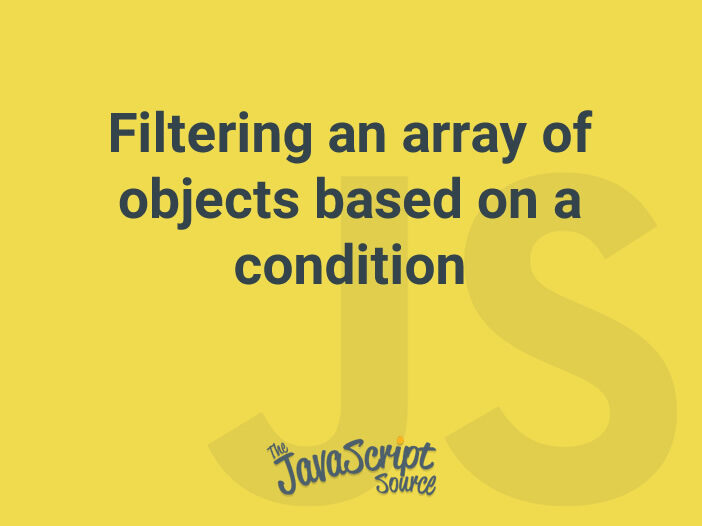
If you have a large array of data and want to filter out items based on a specific condition then you can simply use the filter
function. In this example we have an array of file paths. Some files are in ‘dir1’ while others are in ‘dir2’. Let’s say we want to filter for only a specific directory:
let data = [
"files/dir1/file",
"files/dir1/file2",
"files/dir2/file",
"files/dir2/file2"
];
let filteredData = data.filter(path => path.includes('dir2'));
console.log(filteredData);
--> [ 'files/dir2/file', 'files/dir2/file2' ]
Filtering for a specific directory within the above array of paths is easy. By specifying that the path string must include the string ‘dir2’ you will filter out any paths that don’t contain ‘dir2’ in them. Remember whatever function you pass to filter must return true
for the item to be included in the result.
Source
https://levelup.gitconnected.com/6-javascript-code-snippets-for-solving-common-problems-33deb6cacef3