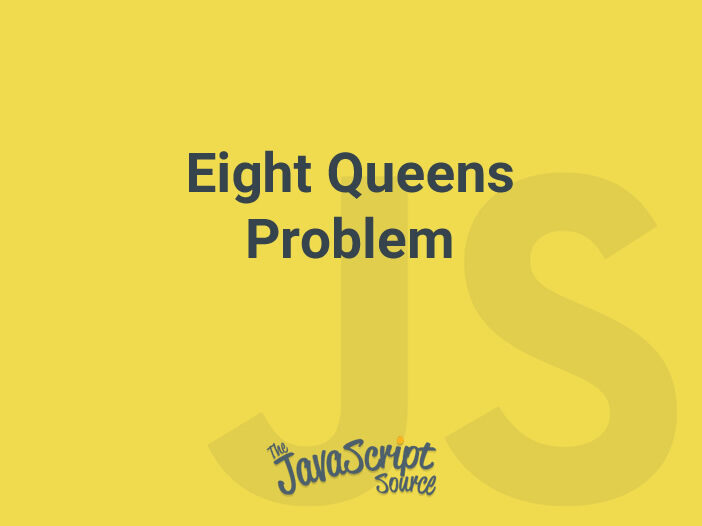
This is a JavaScript that involves the movement of the Queen in Chess. Simply place eight queens on a chess board without conflict.
<!– ONE STEP TO INSTALL EIGHT QUEENS PROBLEM:
1. Copy the coding into the BODY of your HTML document –>
<!– STEP ONE: Paste this code into the BODY of your HTML document –>
<BODY>
<!– This script and many more are available free online at –>
<!– The JavaScript Source!! http://javascript.internet.com –>
<!– Original: Ronald Daenzer ([email protected]) –>
<!– Web Site: http://as400.ferris.edu/daer/EightQueensProb.htm –>
<form name=myform>
<div align=center>
<h1>Eight Queens problem</h1>
Place eight queens on chess board without conflict<br>
<table border=1 width=300 height=300 style=cursor:hand>
<script>
//eight queens problem
//ron daenzer
var closed = new Array(64);
var imgQueen = new Image(32,32);
var imgEmpty = new Image(32,32);
var imgDot = new Image(32,32);
var id=0;
var count=0;
imgQueen.src = “queen.gif”;
imgEmpty.src = “empty.gif”;
imgDot.src = “dot.gif”;
for (var r=0; r<8; r++) //8 rows
{
document.write(‘<tr>’);
for (var c=0; c<8; c++) //8 columns
{
id=(r*8)+c; //calculate cell id
document.write(‘<td id=’+id+’ onclick=”placeq(this);”>’);
document.write(‘<img src=”empty.gif”></td>’);
}
document.write(‘</tr>’);
}
function placeq (cell)
{
var q = eval(cell.id);
var r = Math.floor(q /8);
var c = q % 8;
var min = Math.min(r,c);
var max = Math.max(r,c);
if ( (document.images[q].src == imgEmpty.src) && (safe(q)) )
{
//document.images[q].src = imgQueen.src;
count++;
for (var h=r*8; h<(r*8)+8; h++)
{ closed[h]=h; document.images[h].src=imgDot.src; }
for (var v=c; v<64; v=v+8)
{ closed[v]=v; document.images[v].src=imgDot.src; }
if (c>r) { var x1=c-r; var x2=q+(9*(8-c)); }
else { var x1=(r-c)*8; var x2=q+(9*(8-r)); }
for (var x=x1; x<x2; x=x+9)
{ closed[x]=x; document.images[x].src=imgDot.src; }
if (r+c>7) { var y1=q-((7-c)*7); var y2=q+(7*(8-r)); }
else { var y1=r+c; var y2=q+(8*c); }
for (var y=y1; y<y2; y=y+7)
{ closed[y]=y; document.images[y].src=imgDot.src; }
document.images[q].src = imgQueen.src;
}
if (count == 8)
alert(“Good job, you solved it!”);
if (count > 8)
alert(“Too many queens”);
}
function safe (q)
{ //safe position to place queen on board
if ( closed[q]==q )
return false;
else
return true;
}
function help ()
{ //help print an example of 8 queens
var a = new Array(0,12,23,29,34,46,49,59);
clearBoard();
for (var i=0; i<8; i++)
window.document.images[ a[i] ].src = imgQueen.src;
count=8;
for (var q=0; q<64; q++)
closed[q] = q;
}
function clearBoard ()
{ //clear the image array
for (var i=0; i<64; i++)
window.document.images[i].src = imgEmpty.src;
count=0;
closed = new Array(64);
}
</script>
</table>
<input type=button value=” clear ” onclick=”clearBoard()”>
<input type=button value=” help ” onclick=”help()”>
</div>
</form>
<p><center>
<font face=”arial, helvetica” size”-2″>Free JavaScripts provided<br>
by <a href=”https://javascriptsource.com”>The JavaScript Source</a></font>
</center><p>