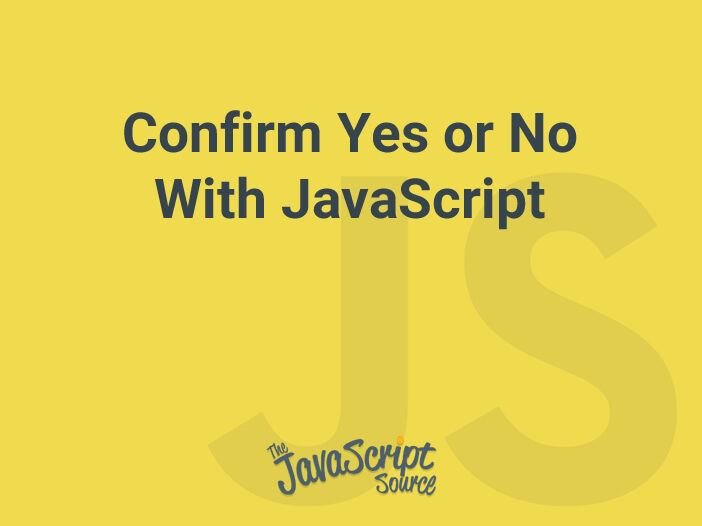
How to display a confirm dialog box using JavaScript. The confirm dialog box allows you to perform actions based on the user input.
In JavaScript, you can use the confirm
method of the window
object to display a dialog box, and wait for the user to either confirm or cancel it. Here’s how it works.
The syntax of the confirm method looks like this:
var result = window.confirm(message);
The confirm method takes a single string argument, and you can pass a message which you want to display in a dialog box. It’s an optional argument, but you’ll want to pass a sensible message—otherwise a blank dialog box with yes and no options will be displayed and probably won’t make any sense to your visitors. Usually, a message is in the form of a question, and a user is presented with two options to choose from.
In a dialog box, there are two buttons: OK and Cancel. If a user clicks on the OK button, the confirm method returns true
, and if a user clicks on the cancel button, the confirm method returns false
. So you can use the return value of the confirm method to know the user’s selection. (If you want the buttons to say something different, like Yes and No, I’ll show you how at the bottom of this post.)
Since the window
object is always implicit, which is to say its properties and methods are always in scope, you can also call the confirm
method, as shown in the following snippet.
var result = confirm(message);
Source
https://code.tutsplus.com/tutorials/confirm-yes-or-no-with-javascript–cms-37532