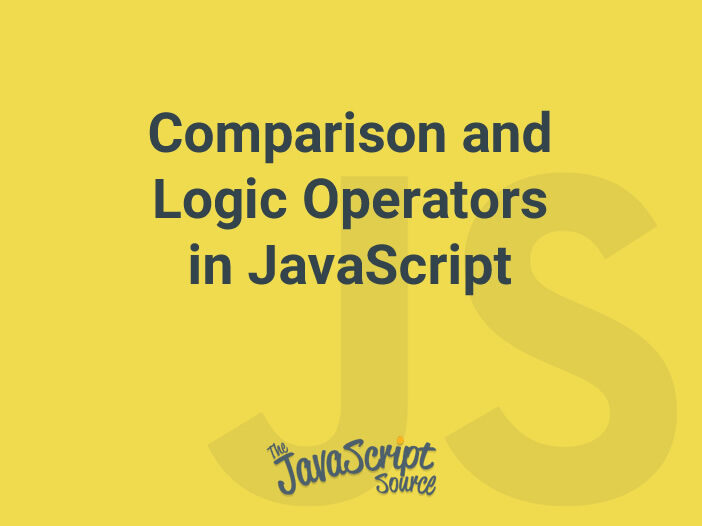
JavaScript has several comparison and logic operators that are used to compare values or perform logical operations. These operators include:
Equality (==)
This operator compares two values for equality. It converts the operands to the same type before making the comparison. For example:
console.log(5 == '5'); // true
console.log(5 === '5'); // false
In the above example, the equality operator (==) returns true because the operands are equal after being converted to the same type (number and string), while the strict equality operator (===) returns false because the operands are not of the same type.
Strict Equality (===)
This operator compares two values for equality without converting the operands to the same type. It is considered to be more strict than the Equality operator. For example:
console.log(5 === 5); // true
console.log(5 === '5'); // false
In this example, the strict equality operator (===) returns true because the operands are of the same type and value, while the second example returns false because the operands are not of the same type.
Inequality (!=)
This operator compares two values for inequality. It converts the operands to the same type before making the comparison. For example:
console.log(5 != '5'); // false
console.log(5 !== '5'); // true
In the above example, the inequality operator (!=) returns false because the operands are equal after being converted to the same type (number and string), while the strict inequality operator (!==) returns true because the operands are not of the same type.
Strict Inequality (!==)
This operator compares two values for inequality without converting the operands to the same type. For example:
console.log(5 !== 5); // false
console.log(5 !== '5'); // true
In this example, the strict inequality operator (!==) returns false because the operands are of the same type and value, while the second example returns true because the operands are not of the same type.
Greater Than (>)
This operator compares if the left operand is greater than the right operand. For example:
console.log(5 > 3); // true
console.log(5 > 5); // false
Less Than (<)
This operator compares if the left operand is less than the right operand. For example:
console.log(3 < 5); // true
console.log(5 < 5); // false
Greater Than or Equal To (>=)
This operator compares if the left operand is greater than or equal to the right operand. For example:
console.log(5 >= 3); // true
console.log(5 >= 5); // true
Less Than or Equal To (<=)
This operator compares if the left operand is less than or equal to the right operand. For example:
console.log(3 <= 5); // true
console.log(5 <= 5); // true
Logical And (&&)
This operator returns true if both operands are true, and false otherwise. For example:
console.log(true && true); // true
console.log
Logical Or (||)
This operator returns true if at least one of the operands is true, and false if both operands are false. For example:
console.log(true || true); // true
console.log(true || false); // true
console.log(false || false); // false
Logical Not (!)
This operator inverts the value of the operand. For example:
console.log(!true); // false
console.log(!false); // true
Ternary Operator (a ? b : c)
This operator is a shorthand for an if-else statement. It evaluates the expression before the question mark and if it’s true, it returns the value before the colon, otherwise it returns the value after the colon. For example:
let x = 10;
let y = x > 5 ? "x is greater than 5" : "x is less than or equal to 5";
console.log(y); // "x is greater than 5"
It’s important to note that the order of operations, such as the order of comparison operators and logical operators, can affect the outcome of a statement. To ensure that your code is working as intended, it is recommended to use parentheses to explicitly define the order of operations.